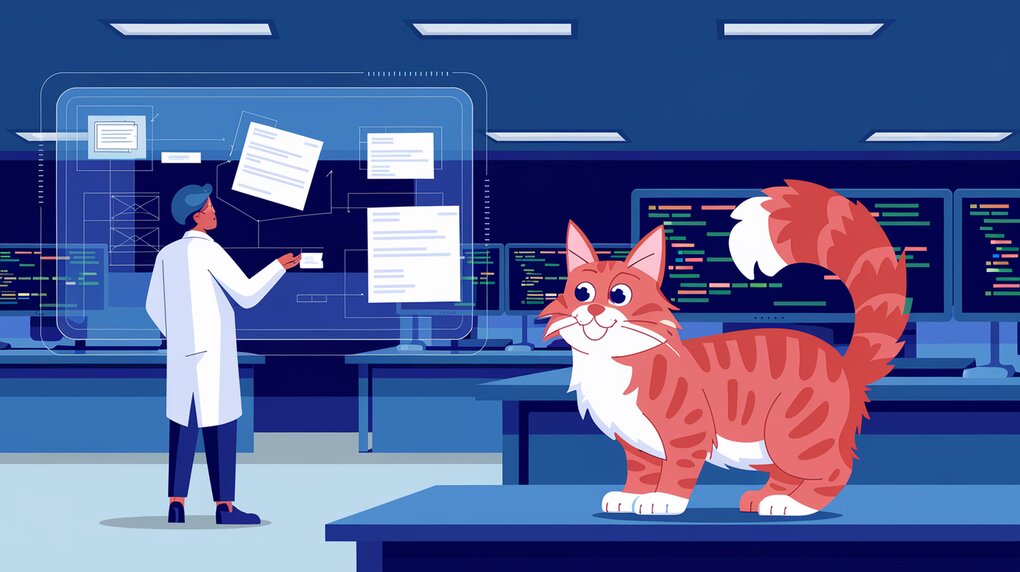
Introducing Uniforms: The Schema-Driven Form Builder for React
In the ever-evolving landscape of React development, creating forms can often be a tedious and repetitive task. Enter uniforms, a set of React libraries designed to streamline the process of building forms from any schema. This powerful tool offers developers a unique approach to form creation, combining automation with flexibility to enhance productivity and maintain consistency across projects.
Core Features: What Sets Uniforms Apart
Uniforms boasts an impressive array of features that make it stand out in the crowded field of form libraries:
-
Automatic Form Generation: Perhaps the most compelling feature, uniforms can completely generate forms based on your schema, eliminating the need for manual field creation.
-
Schema Flexibility: The library supports various schema types, including JSON Schema, GraphQL, and SimpleSchema, allowing developers to work with their preferred data description format.
-
Custom Field Creation: Uniforms provides a straightforward helper for creating custom fields, enabling developers to extend functionality with minimal effort.
-
Robust Validation: The library offers both inline and asynchronous form validation, ensuring data integrity at every step.
-
Theme Support: With a wide range of theme options, uniforms integrates seamlessly with popular UI frameworks like Semantic UI, Material-UI, and Bootstrap.
Getting Started with Uniforms
To begin your journey with uniforms, you’ll need to install three key packages:
npm install uniforms uniforms-bridge-json-schema uniforms-semantic
This setup includes the core uniforms package, a bridge for JSON Schema (you can choose alternatives based on your schema type), and a theme package (in this case, Semantic UI).
Creating Your First Uniforms Form
Let’s create a simple guest registration form for an IT conference to demonstrate uniforms in action:
import Ajv, { JSONSchemaType } from 'ajv';
import { JSONSchemaBridge } from 'uniforms-bridge-json-schema';
import { AutoForm } from 'uniforms-semantic';
// Define the form data structure
type FormData = {
firstName: string;
lastName: string;
workExperience: number;
};
// Create the schema
const schema: JSONSchemaType<FormData> = {
title: 'Guest',
type: 'object',
properties: {
firstName: { type: 'string' },
lastName: { type: 'string' },
workExperience: {
description: 'Work experience in years',
type: 'integer',
minimum: 0,
maximum: 100,
},
},
required: ['firstName', 'lastName'],
};
// Set up the validator
const ajv = new Ajv({ allErrors: true, useDefaults: true, keywords: ['uniforms'] });
const validator = ajv.compile(schema);
// Create the bridge
const bridge = new JSONSchemaBridge({
schema,
validator: (model: Record<string, unknown>) => {
validator(model);
return validator.errors?.length ? { details: validator.errors } : null;
},
});
// Render the form
function GuestForm() {
return <AutoForm schema={bridge} onSubmit={console.log} />;
}
This concise code snippet generates a fully functional form with labeled fields, validation, and a submit button. The AutoForm
component from uniforms takes care of the heavy lifting, interpreting the schema and creating the appropriate form elements.
Advanced Usage and Customization
While the automatic form generation is impressive, uniforms truly shines in its flexibility for customization:
Custom Fields
You can create custom fields to handle specific data types or complex input requirements:
import { connectField } from 'uniforms';
const CustomField = connectField(({ onChange, value }) => (
<input
onChange={event => onChange(event.target.value)}
value={value}
/>
));
Conditional Fields
Implement dynamic form behavior based on user input:
function ConditionalForm() {
return (
<AutoForm
schema={bridge}
onSubmit={console.log}
>
<AutoField name="isStudent" />
{model => model.isStudent && <AutoField name="studentId" />}
</AutoForm>
);
}
Asynchronous Validation
Handle complex validation scenarios with asynchronous validators:
const asyncValidator = model =>
new Promise(resolve => {
setTimeout(() => {
const errors = [];
if (model.email.includes('test')) {
errors.push({ name: 'email', message: 'Test emails not allowed' });
}
resolve(errors.length ? { details: errors } : null);
}, 1000);
});
const bridge = new JSONSchemaBridge({ schema, validator: asyncValidator });
Performance Considerations
Uniforms is designed with performance in mind, utilizing React’s reconciliation process efficiently. However, for extremely large forms or complex schemas, consider breaking the form into smaller sections or implementing virtualization techniques.
Community and Ecosystem
The uniforms library benefits from an active community and comprehensive documentation. With over 2,000 stars on GitHub and regular updates, it’s a well-maintained project that continues to evolve with the React ecosystem.
Conclusion: Is Uniforms Right for Your Project?
Uniforms offers a compelling solution for React developers looking to streamline their form creation process. Its ability to generate forms automatically from schemas can significantly reduce development time and ensure consistency across large projects.
For teams working with complex data structures or those requiring highly customizable forms, uniforms provides a robust foundation. Its integration with popular UI frameworks and support for various schema types make it a versatile choice for a wide range of applications.
However, for simple forms or projects with very specific custom requirements, the overhead of setting up uniforms might outweigh its benefits. As with any tool, it’s essential to evaluate uniforms in the context of your project’s specific needs.
In conclusion, if you’re dealing with data-driven forms in React and want to boost your productivity without sacrificing flexibility, uniforms is definitely worth considering. Its schema-first approach and rich feature set make it a powerful ally in the quest for efficient and maintainable form development.