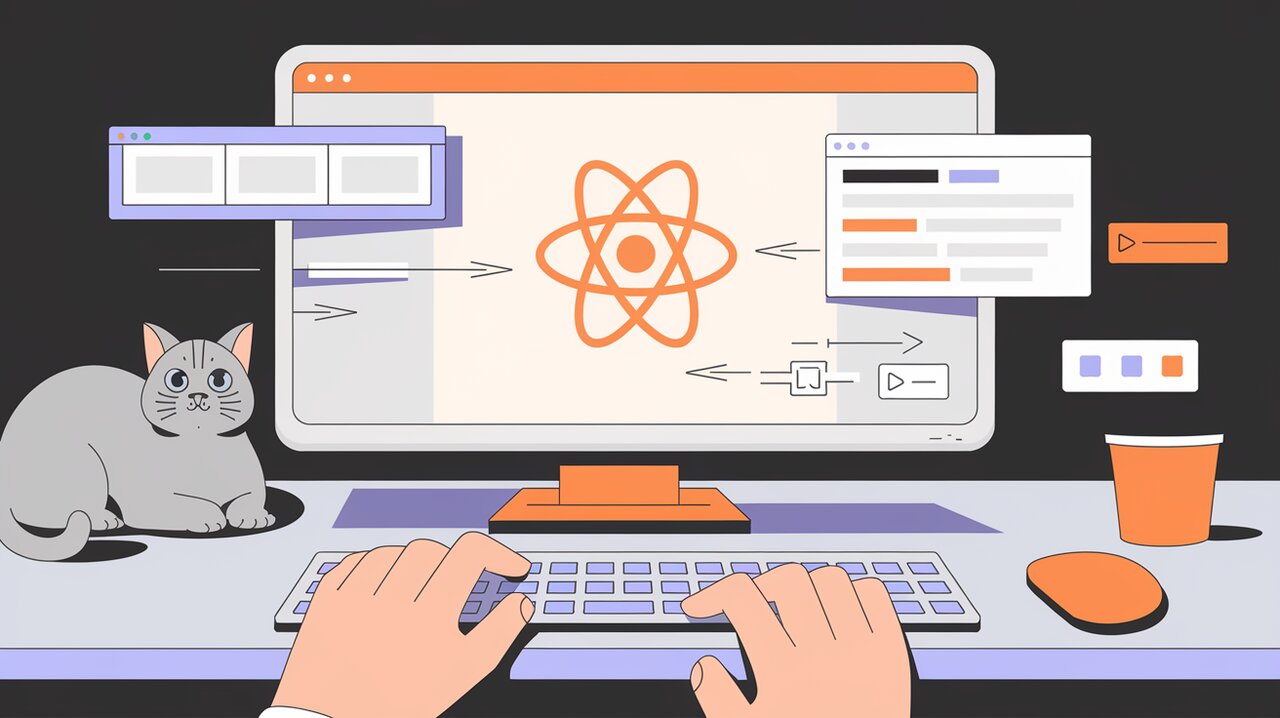
Unleash the Power of Click-Outside Detection with react-cool-onclickoutside
React developers often face the challenge of managing UI interactions, particularly when it comes to closing dropdowns, modals, or tooltips when a user clicks outside of them. Enter react-cool-onclickoutside, a powerful and lightweight hook that simplifies this common task, allowing developers to create more intuitive and responsive user interfaces with ease.
Features
react-cool-onclickoutside
comes packed with an impressive array of features that make it a standout solution for handling click-outside events:
- Hook-based implementation for modern React applications
- Support for multiple refs to cover complex use cases
- Passive event listeners for improved scrolling performance
- Option to exclude scrollbar from outside click detection
- Ability to ignore specific elements during event handling
- Flexible event listener management
- iframe click detection for enhanced developer experience
- Custom ref support for specialized scenarios
- TypeScript compatibility
- Server-side rendering friendly
- Minimal bundle size impact (< 1kB gzipped)
Installation
Getting started with react-cool-onclickoutside
is straightforward. You can install it using either npm or yarn:
# Using npm
npm install react-cool-onclickoutside
# Using yarn
yarn add react-cool-onclickoutside
Basic Usage
Let’s dive into a common use case to demonstrate how react-cool-onclickoutside
can simplify your code and improve user experience.
Creating a Dismissible Dropdown
Here’s an example of how to create a dropdown menu that closes when the user clicks outside of it:
import React, { useState } from 'react';
import useOnclickOutside from 'react-cool-onclickoutside';
const Dropdown: React.FC = () => {
const [isOpen, setIsOpen] = useState(false);
const ref = useOnclickOutside(() => {
setIsOpen(false);
});
const toggleDropdown = () => {
setIsOpen(!isOpen);
};
return (
<div>
<button onClick={toggleDropdown}>Toggle Dropdown</button>
{isOpen && (
<div ref={ref}>
<ul>
<li>Option 1</li>
<li>Option 2</li>
<li>Option 3</li>
</ul>
</div>
)}
</div>
);
};
In this example, the useOnclickOutside
hook is used to create a ref that’s attached to the dropdown menu. When a click occurs outside of this ref, the callback function is triggered, closing the dropdown.
Handling Multiple Elements
One of the strengths of react-cool-onclickoutside
is its ability to handle multiple elements simultaneously. This is particularly useful when you have complex UI components that need to be dismissed together.
import React, { useState } from 'react';
import useOnclickOutside from 'react-cool-onclickoutside';
const ComplexUI: React.FC = () => {
const [isVisible, setIsVisible] = useState(true);
const ref = useOnclickOutside(() => {
setIsVisible(false);
});
return (
<div>
{isVisible && (
<>
<div ref={ref}>Tooltip 1</div>
<div ref={ref}>Tooltip 2</div>
</>
)}
<button onClick={() => setIsVisible(true)}>Show Tooltips</button>
</div>
);
};
In this case, both tooltips will be dismissed when clicking outside of either of them, as they share the same ref.
Advanced Usage
react-cool-onclickoutside
offers several advanced features that cater to more complex scenarios. Let’s explore some of these capabilities.
Ignoring Specific Elements
There are times when you want to ignore clicks on certain elements, even if they’re outside your target component. The ignore
option allows you to achieve this:
import React, { useState } from 'react';
import useOnclickOutside from 'react-cool-onclickoutside';
const AdvancedDropdown: React.FC = () => {
const [isOpen, setIsOpen] = useState(false);
const ref = useOnclickOutside(
() => {
setIsOpen(false);
},
{ ignore: ['.ignore-me'] }
);
return (
<div>
<button onClick={() => setIsOpen(!isOpen)}>Toggle Dropdown</button>
{isOpen && (
<div ref={ref}>
<ul>
<li>Option 1</li>
<li>Option 2</li>
</ul>
</div>
)}
<button className="ignore-me">This won't close the dropdown</button>
</div>
);
};
Disabling the Event Listener
For performance reasons or specific use cases, you might want to temporarily disable the outside click detection. The disabled
option provides this functionality:
import React, { useState } from 'react';
import useOnclickOutside from 'react-cool-onclickoutside';
const DisableableComponent: React.FC = () => {
const [isActive, setIsActive] = useState(true);
const [content, setContent] = useState('Click outside to hide me');
const ref = useOnclickOutside(
() => {
setContent('Hidden!');
},
{ disabled: !isActive }
);
return (
<div>
<div ref={ref}>{content}</div>
<button onClick={() => setIsActive(!isActive)}>
{isActive ? 'Disable' : 'Enable'} outside click
</button>
</div>
);
};
Detecting iframe Clicks
Working with iframes can be tricky, but react-cool-onclickoutside
has you covered. It can detect clicks within iframes, ensuring a seamless experience across different document contexts:
import React, { useState } from 'react';
import useOnclickOutside from 'react-cool-onclickoutside';
const IframeAwareComponent: React.FC = () => {
const [message, setMessage] = useState('Interact to see behavior');
const ref = useOnclickOutside(
() => {
setMessage('Clicked outside, including iframe content!');
},
{ detectIframe: true }
);
return (
<div ref={ref}>
<p>{message}</p>
<iframe src="https://example.com" title="Example Content" />
</div>
);
};
Conclusion
react-cool-onclickoutside
is a powerful tool in the React developer’s arsenal, offering a clean and efficient way to handle click-outside events. Its flexibility, performance optimizations, and thoughtful features make it an excellent choice for creating responsive and user-friendly interfaces.
By leveraging this hook, developers can significantly reduce boilerplate code and focus on crafting exceptional user experiences. Whether you’re building simple dropdowns or complex modal systems, react-cool-onclickoutside
provides the functionality you need with minimal overhead.
As you continue to develop React applications, consider incorporating react-cool-onclickoutside
into your toolkit. It’s a small addition that can make a big difference in how your users interact with your UI components, leading to more polished and professional web applications.