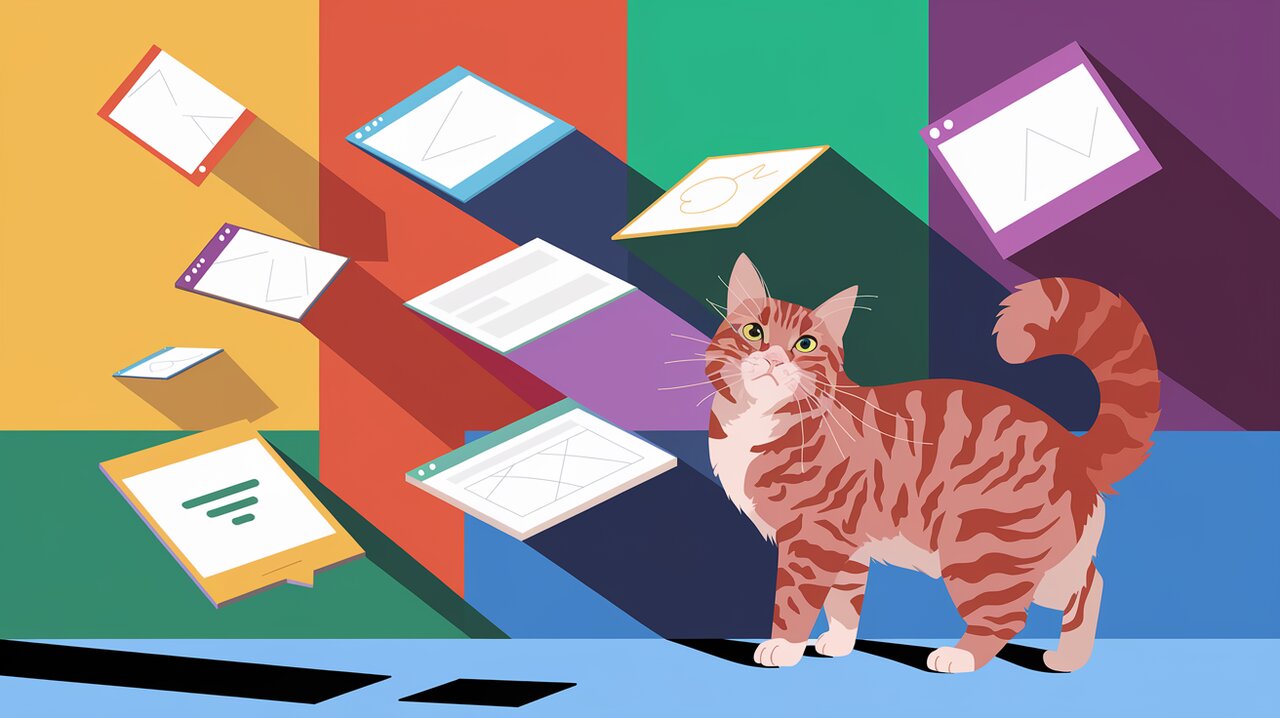
Unleash the Power of Drag and Drop with React-Draggable
Empowering Your React Applications with Drag and Drop
In the world of modern web development, creating interactive and engaging user interfaces is paramount. One of the most sought-after features is the ability to drag and drop elements within a web application. Enter React-Draggable, a powerful and flexible library that brings this functionality to your React projects with ease.
React-Draggable is a simple yet robust component that allows you to make any element draggable within your React application. Whether you’re building a complex dashboard, a customizable user interface, or just want to add some interactivity to your components, this library has got you covered.
Key Features of React-Draggable
React-Draggable comes packed with a variety of features that make it a go-to choice for developers:
- Simplicity: With a straightforward API, implementing drag and drop functionality becomes a breeze.
- Flexibility: The library works with both mouse and touch events, ensuring compatibility across devices.
- Customization: Developers can easily control drag behavior, set boundaries, and customize the drag handle.
- Performance: Built with efficiency in mind, it ensures smooth dragging even in complex applications.
- TypeScript Support: Full TypeScript definitions are included, making it easy to use in TypeScript projects.
Getting Started with React-Draggable
Installation
To start using React-Draggable in your project, you first need to install it. You can do this using either npm or yarn:
npm install react-draggable
Or if you prefer yarn:
yarn add react-draggable
Basic Usage
Once installed, you can start using React-Draggable in your React components. Here’s a simple example to get you started:
import React from 'react';
import Draggable from 'react-draggable';
const DraggableComponent: React.FC = () => {
return (
<Draggable>
<div>I can be dragged anywhere!</div>
</Draggable>
);
};
export default DraggableComponent;
In this basic example, we import the Draggable
component from react-draggable
and wrap our div with it. This immediately makes the div draggable within its parent container.
Controlling Drag Behavior
React-Draggable allows you to fine-tune the drag behavior of your elements. Here’s an example that demonstrates how to set axis constraints and initial position:
import React from 'react';
import Draggable from 'react-draggable';
const ControlledDraggable: React.FC = () => {
return (
<Draggable
axis="x"
defaultPosition={{x: 0, y: 0}}
grid={[25, 25]}
>
<div>I can only be dragged horizontally, and I snap to a 25x25 grid!</div>
</Draggable>
);
};
export default ControlledDraggable;
In this example, we’ve added several props to the Draggable
component:
axis="x"
restricts movement to the horizontal axis only.defaultPosition
sets the initial position of the element.grid
makes the element snap to a 25x25 pixel grid as it’s dragged.
Advanced Techniques with React-Draggable
Handling Drag Events
React-Draggable provides a set of event handlers that allow you to respond to various stages of the drag operation. Here’s an example that logs the drag start and end positions:
import React from 'react';
import Draggable, { DraggableEvent, DraggableData } from 'react-draggable';
const EventHandlingDraggable: React.FC = () => {
const handleStart = (e: DraggableEvent, data: DraggableData) => {
console.log('Drag started:', data.x, data.y);
};
const handleStop = (e: DraggableEvent, data: DraggableData) => {
console.log('Drag ended:', data.x, data.y);
};
return (
<Draggable onStart={handleStart} onStop={handleStop}>
<div>Drag me and check the console!</div>
</Draggable>
);
};
export default EventHandlingDraggable;
This example demonstrates how to use the onStart
and onStop
event handlers to log the position of the draggable element at the beginning and end of a drag operation.
Creating a Custom Drag Handle
Sometimes, you may want only a specific part of your component to be draggable. React-Draggable makes this easy with the handle
prop:
import React from 'react';
import Draggable from 'react-draggable';
const CustomHandleDraggable: React.FC = () => {
return (
<Draggable handle=".handle">
<div style={{ border: '1px solid black', padding: '10px' }}>
<div className="handle" style={{ background: 'gray', padding: '5px' }}>Drag here</div>
<p>This content is not draggable</p>
</div>
</Draggable>
);
};
export default CustomHandleDraggable;
In this example, only the element with the class “handle” can be used to drag the entire component.
Implementing Bounds
To restrict the draggable area, you can use the bounds
prop. This is particularly useful when you want to keep the draggable element within a specific container:
import React from 'react';
import Draggable from 'react-draggable';
const BoundedDraggable: React.FC = () => {
return (
<div style={{ height: '300px', width: '300px', border: '1px solid black' }}>
<Draggable bounds="parent">
<div style={{ padding: '10px', background: 'lightblue' }}>
I can't escape my parent container!
</div>
</Draggable>
</div>
);
};
export default BoundedDraggable;
This example creates a draggable element that is confined within its parent div, preventing it from being dragged outside the defined boundaries.
Wrapping Up Your Drag and Drop Journey
React-Draggable opens up a world of possibilities for creating interactive and dynamic user interfaces in your React applications. From simple draggable elements to complex, bounded, and event-driven components, this library provides the tools you need to enhance user experience and engagement.
By mastering the features and techniques outlined in this article, you’ll be well-equipped to implement drag and drop functionality that not only meets your project requirements but also delights your users. Remember, the key to creating great interactive elements is experimentation and iteration. So, don’t hesitate to play around with different configurations and see what works best for your specific use case.
As you continue to explore the capabilities of React-Draggable, you’ll discover even more ways to leverage its power in your projects. Happy dragging!