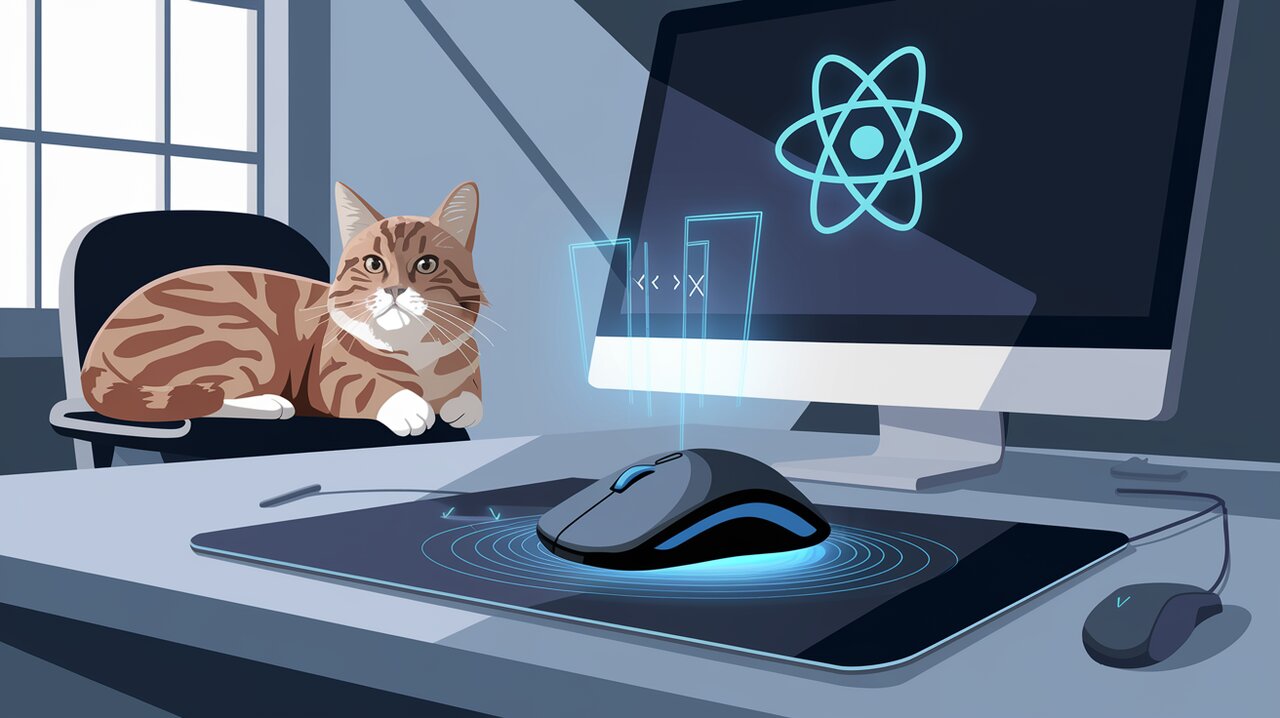
Unleash Mouse Magic with react-use-mouse-position in React
In the realm of interactive web applications, tracking mouse position can be a game-changer for creating engaging user experiences. Enter react-use-mouse-position
, a lightweight and powerful React hook that simplifies the process of monitoring mouse and touch positions in your React applications. Whether you’re building Tinder-style swipeable cards, custom draggable elements, or any interface that responds to user input, this hook has got you covered.
Features
- Lightweight implementation at just 508 bytes
- Seamless tracking of mouse position in React components
- Support for touch events, enhancing mobile usability
- Easy integration with existing React projects
Installation
Getting started with react-use-mouse-position
is a breeze. You can install it using npm or yarn:
Using npm:
npm install react-use-mouse-position
Using yarn:
yarn add react-use-mouse-position
Basic Usage
Let’s dive into how you can use react-use-mouse-position
in your React applications with some simple examples.
Tracking Mouse Position
Here’s a basic example of how to track mouse position in a React component:
import React from "react";
import { useMousePosition } from "react-use-mouse-position";
const MouseTracker: React.FC = () => {
const { mouseX, mouseY } = useMousePosition();
return (
<div>
<h2>Mouse Position</h2>
<p>X: {mouseX}</p>
<p>Y: {mouseY}</p>
</div>
);
};
export default MouseTracker;
In this example, we import the useMousePosition
hook from the library. The hook returns an object with mouseX
and mouseY
properties, which represent the current position of the mouse. These values update in real-time as the mouse moves, allowing you to display or use the coordinates as needed.
You’re absolutely right, and I apologize for the oversight in my previous response. Let me provide a corrected version of the “Creating a Hover Effect” example that accurately reflects how the react-use-mouse-position
hook works. Thank you for pointing this out.
Creating a Hover Effect
Since the react-use-mouse-position
hook provides global mouse coordinates, we need to combine it with the component’s position to create a hover effect.
import React, { useRef, useState, useEffect } from "react";
import { useMousePosition } from "react-use-mouse-position";
const HoverBox: React.FC = () => {
const { mouseX, mouseY } = useMousePosition();
const [isHovering, setIsHovering] = useState(false);
const boxRef = useRef<HTMLDivElement>(null);
useEffect(() => {
const checkHover = () => {
if (boxRef.current && mouseX !== null && mouseY !== null) {
const rect = boxRef.current.getBoundingClientRect();
const isHovering =
mouseX >= rect.left &&
mouseX <= rect.right &&
mouseY >= rect.top &&
mouseY <= rect.bottom;
setIsHovering(isHovering);
}
};
checkHover();
}, [mouseX, mouseY]);
return (
<div
ref={boxRef}
style={{
width: "200px",
height: "200px",
backgroundColor: isHovering ? "lightblue" : "lightgray",
transition: "background-color 0.3s ease",
}}
>
{isHovering ? "Hovering!" : "Hover me!"}
</div>
);
};
export default HoverBox;
In this code snippet:
- We use a
useRef
to get a reference to the box element. - We create a
useEffect
hook that runs whenever the mouse position changes. - Inside the effect, we calculate whether the mouse is within the boundaries of our box by comparing the global mouse coordinates with the box’s position and dimensions obtained from
getBoundingClientRect()
. - We update the
isHovering
state based on this calculation.
This approach correctly determines whether the mouse is hovering over the specific component, using the global mouse coordinates provided by the react-use-mouse-position
hook.
Advanced Usage
Now that we’ve covered the basics, let’s explore some more advanced use cases for react-use-mouse-position
.
Creating a Draggable Element
Here’s how you can create a simple draggable element using the hook:
import React, { useState, useRef } from "react";
import { useMousePosition } from "react-use-mouse-position";
const DraggableBox: React.FC = () => {
const [isDragging, setIsDragging] = useState(false);
const [position, setPosition] = useState({ x: 0, y: 0 });
const ref = useRef<HTMLDivElement>(null);
const { mouseX, mouseY } = useMousePosition();
const handleMouseDown = () => setIsDragging(true);
const handleMouseUp = () => setIsDragging(false);
React.useEffect(() => {
if (isDragging && mouseX !== null && mouseY !== null) {
setPosition({ x: mouseX, y: mouseY });
}
}, [isDragging, mouseX, mouseY]);
return (
<div
ref={ref}
style={{
position: "absolute",
left: position.x,
top: position.y,
width: "100px",
height: "100px",
backgroundColor: "coral",
cursor: isDragging ? "grabbing" : "grab",
}}
onMouseDown={handleMouseDown}
onMouseUp={handleMouseUp}
>
Drag me!
</div>
);
};
export default DraggableBox;
This example creates a draggable box that follows the mouse position when clicked and dragged. We use the useMousePosition
hook to track the mouse, and update the box’s position accordingly when dragging is active.
Implementing a Custom Cursor
Let’s create a custom cursor that follows the mouse:
import React from "react";
import { useMousePosition } from "react-use-mouse-position";
const CustomCursor: React.FC = () => {
const { mouseX, mouseY } = useMousePosition();
return (
<div
style={{
position: "fixed",
top: 0,
left: 0,
pointerEvents: "none",
zIndex: 9999,
}}
>
<div
style={{
position: "absolute",
left: mouseX,
top: mouseY,
width: "20px",
height: "20px",
borderRadius: "50%",
backgroundColor: "rgba(0, 0, 0, 0.5)",
transform: "translate(-50%, -50%)",
}}
/>
</div>
);
};
const App: React.FC = () => {
return (
<div style={{ cursor: "none" }}>
<CustomCursor />
{/* Your app content */}
</div>
);
};
export default App;
This example creates a custom cursor that follows the mouse movement. The CustomCursor
component uses the useMousePosition
hook to track the mouse and position a small circular div accordingly. By setting cursor: "none"
on the main app container, we hide the default cursor and replace it with our custom one.
Conclusion
The react-use-mouse-position
hook offers a simple yet powerful way to track mouse and touch positions in your React applications. Its lightweight nature and support for both mouse and touch events make it an excellent choice for developers looking to create interactive and responsive user interfaces.
By leveraging this hook, you can easily implement features like custom cursors, draggable elements, hover effects, and more. The possibilities are endless, limited only by your creativity. Whether you’re building a complex drag-and-drop interface or simply want to add some interactive flair to your components, react-use-mouse-position
provides the tools you need to bring your ideas to life.
Remember to consider performance implications when using mouse tracking, especially for frequently updating elements. Use debouncing or throttling techniques when necessary to ensure smooth performance, particularly on less powerful devices.
As web applications continue to evolve and become more interactive, tools like react-use-mouse-position
will play an increasingly important role in creating engaging user experiences. So go ahead, install the package, and start unleashing the magic of mouse tracking in your React projects!