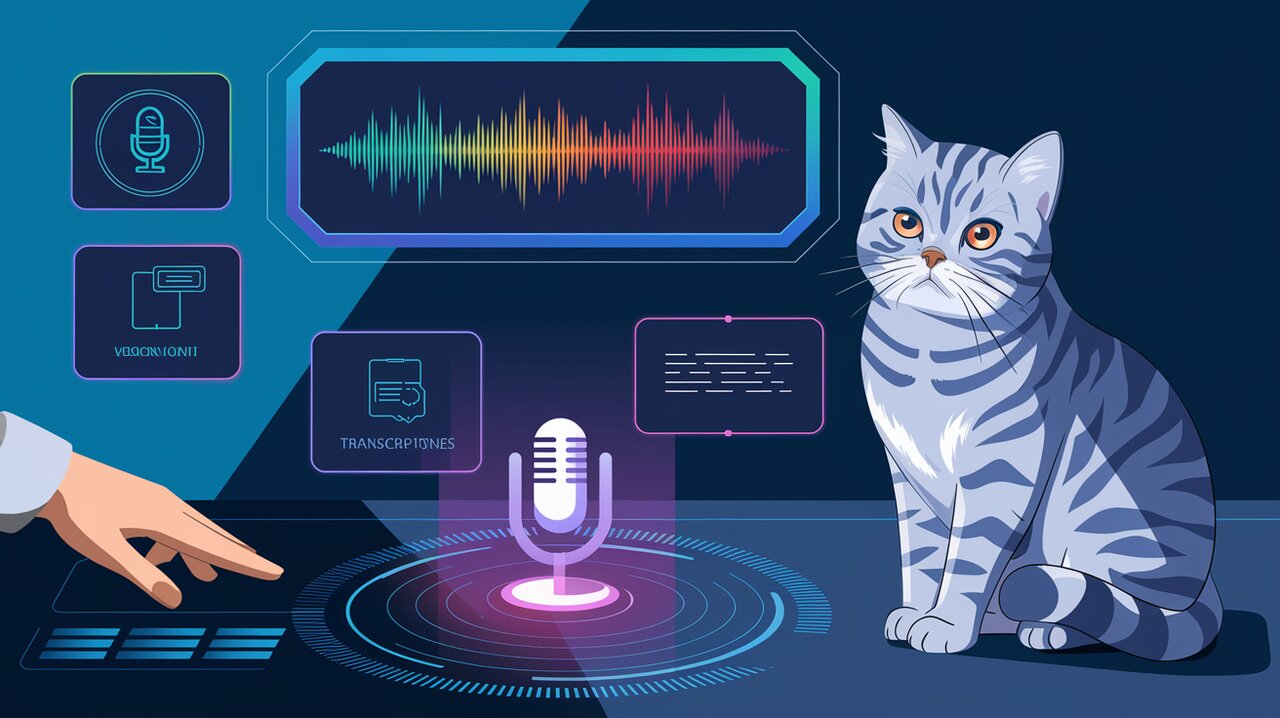
Unleash the Power of Voice: React Speech Recognition Demystified
React Speech Recognition is a powerful library that brings voice input capabilities to your React applications. By leveraging the Web Speech API, it allows developers to easily implement speech-to-text functionality, enhancing user interaction and accessibility. In this article, we’ll explore how to use react-speech-recognition
to create voice-enabled features in your React projects.
Features
react-speech-recognition
offers several key features that make it an excellent choice for adding speech recognition to your React applications:
- Easy integration with React hooks
- Real-time speech-to-text conversion
- Support for continuous listening
- Customizable voice commands
- Cross-browser compatibility (with polyfills)
- TypeScript support
Installation
To get started with react-speech-recognition
, you’ll need to install it in your React project. You can do this using npm or yarn:
npm install react-speech-recognition
# or
yarn add react-speech-recognition
Basic Usage
Let’s dive into a simple example of how to use react-speech-recognition
in your React component:
Creating a Basic Dictaphone
import React from 'react';
import SpeechRecognition, { useSpeechRecognition } from 'react-speech-recognition';
const Dictaphone: React.FC = () => {
const {
transcript,
listening,
resetTranscript,
browserSupportsSpeechRecognition
} = useSpeechRecognition();
if (!browserSupportsSpeechRecognition) {
return <span>Browser doesn't support speech recognition.</span>;
}
return (
<div>
<p>Microphone: {listening ? 'on' : 'off'}</p>
<button onClick={() => SpeechRecognition.startListening()}>Start</button>
<button onClick={SpeechRecognition.stopListening}>Stop</button>
<button onClick={resetTranscript}>Reset</button>
<p>{transcript}</p>
</div>
);
};
export default Dictaphone;
This example demonstrates the basic functionality of react-speech-recognition
. The useSpeechRecognition
hook provides us with the transcript
, listening
state, and functions to control the speech recognition process.
Understanding the Key Components
The useSpeechRecognition
hook returns several useful properties:
transcript
: The current speech-to-text resultlistening
: A boolean indicating whether the microphone is activeresetTranscript
: A function to clear the current transcriptbrowserSupportsSpeechRecognition
: A boolean indicating browser support for speech recognition
The SpeechRecognition
object provides methods to control the recognition process:
startListening()
: Begins the speech recognitionstopListening()
: Stops the speech recognitionabortListening()
: Immediately stops the speech recognition
Advanced Usage
Now that we’ve covered the basics, let’s explore some more advanced features of react-speech-recognition
.
Continuous Listening
For applications that require ongoing voice input, you can enable continuous listening:
const startListening = () => SpeechRecognition.startListening({ continuous: true });
Language Support
You can specify the language for recognition:
SpeechRecognition.startListening({ language: 'fr-FR' });
Implementing Voice Commands
react-speech-recognition
allows you to define custom voice commands:
import React from 'react';
import SpeechRecognition, { useSpeechRecognition } from 'react-speech-recognition';
const VoiceCommands: React.FC = () => {
const commands = [
{
command: 'open *',
callback: (website: string) => window.open('http://' + website)
},
{
command: 'change background color to *',
callback: (color: string) => document.body.style.background = color
}
];
const { transcript } = useSpeechRecognition({ commands });
return (
<div>
<p>Try saying: "open react.js.org" or "change background color to yellow"</p>
<p>{transcript}</p>
</div>
);
};
export default VoiceCommands;
This example demonstrates how to set up voice commands that can open websites or change the background color based on spoken instructions.
Cross-Browser Compatibility
While react-speech-recognition
works best with Chrome, you can use polyfills to ensure cross-browser compatibility. Here’s an example using the Speechly polyfill:
import React from 'react';
import { createSpeechlySpeechRecognition } from '@speechly/speech-recognition-polyfill';
import SpeechRecognition, { useSpeechRecognition } from 'react-speech-recognition';
const appId = '<YOUR_SPEECHLY_APP_ID>';
const SpeechlySpeechRecognition = createSpeechlySpeechRecognition(appId);
SpeechRecognition.applyPolyfill(SpeechlySpeechRecognition);
const CrossBrowserDictaphone: React.FC = () => {
const { transcript, listening, browserSupportsSpeechRecognition } = useSpeechRecognition();
if (!browserSupportsSpeechRecognition) {
return <span>Browser doesn't support speech recognition.</span>;
}
return (
<div>
<p>Microphone: {listening ? 'on' : 'off'}</p>
<button onClick={() => SpeechRecognition.startListening({ continuous: true })}>Start</button>
<button onClick={SpeechRecognition.stopListening}>Stop</button>
<p>{transcript}</p>
</div>
);
};
export default CrossBrowserDictaphone;
This setup ensures that your speech recognition functionality works across different browsers by using the Speechly polyfill.
Conclusion
react-speech-recognition
opens up a world of possibilities for creating voice-enabled React applications. From simple dictation features to complex voice command systems, this library provides the tools you need to enhance user interaction through speech.
By implementing speech recognition in your React apps, you can improve accessibility, create hands-free interfaces, and offer innovative ways for users to interact with your application. As voice technology continues to evolve, integrating these features can give your app a competitive edge and provide a more natural and intuitive user experience.
Remember to consider privacy concerns and always obtain user consent before activating speech recognition features. With the right implementation, react-speech-recognition
can significantly enhance the functionality and user experience of your React applications.