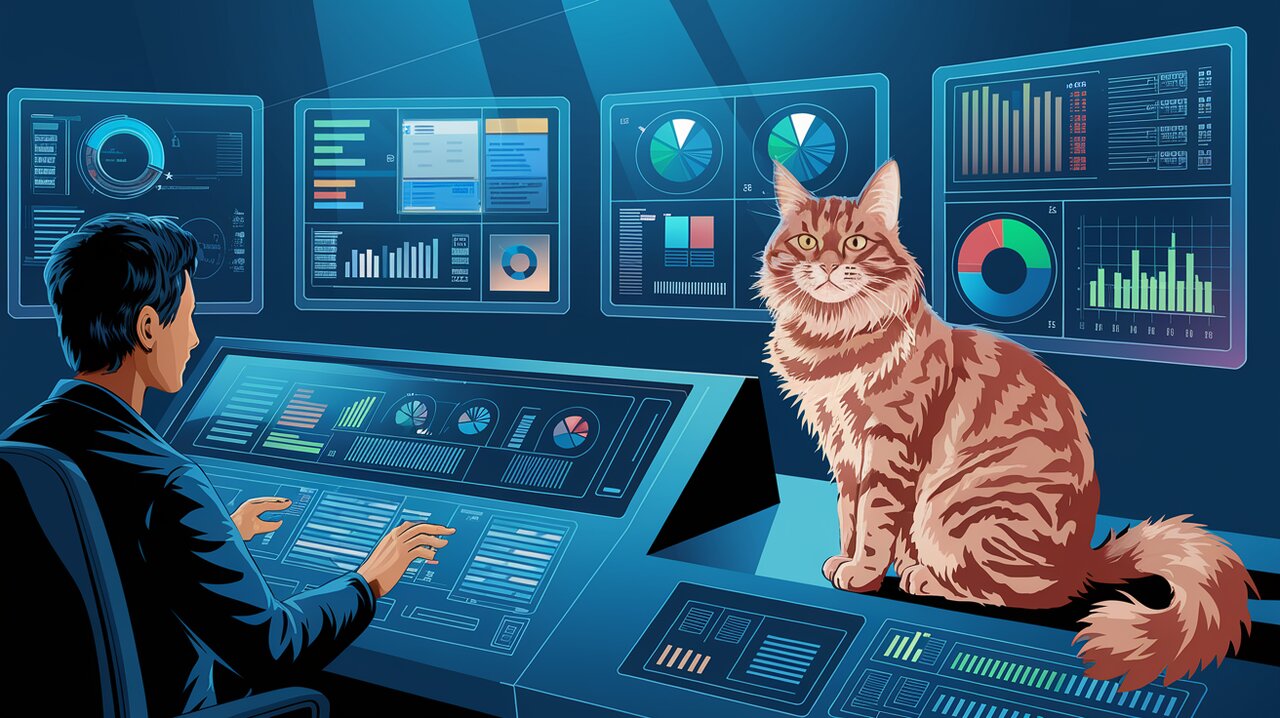
Unleashing Composite Filters: Taming Data with React Filter Control
React Filter Control is a powerful React component designed to help developers create sophisticated composite filter criteria for their applications. This versatile library allows users to build complex filter structures, enabling precise data filtering across various fields and conditions. Whether you’re working on a data-heavy dashboard or a searchable product catalog, React Filter Control provides the tools to enhance your user’s data exploration experience.
Key Features of React Filter Control
React Filter Control comes packed with a range of features that make it a standout choice for implementing advanced filtering in React applications:
- Composite Filtering: Create complex filter structures with nested conditions and groups.
- Customizable Fields: Define custom fields with specific operators tailored to your data types.
- Flexible Operators: Implement a wide range of comparison operators for precise filtering.
- Group-based Filtering: Organize filters into logical groups for better structure and readability.
- TypeScript Support: Enjoy full TypeScript definitions for enhanced development experience.
Getting Started with React Filter Control
Before diving into the implementation details, let’s set up React Filter Control in your project.
Setting Up Your Filtering Arsenal
To begin your journey with React Filter Control, you’ll need to install the package. Open your terminal and run one of the following commands:
npm install react-filter-control
Or if you prefer yarn:
yarn add react-filter-control
With the library installed, you’re ready to start building powerful filter interfaces in your React applications.
Crafting Your First Filter
Let’s create a basic filter control to get a feel for how React Filter Control works.
The Filter Foundation
Here’s a simple example to get you started:
import React from 'react';
import FilterControl from 'react-filter-control';
const fields = [
{ name: 'name', caption: 'Name', operators: [{ name: 'contains', caption: 'Contains' }] },
{ name: 'age', caption: 'Age', operators: [{ name: 'gte', caption: 'Greater than or equal' }] }
];
const initialFilterValue = {
groupName: 'and',
items: []
};
const App: React.FC = () => {
const [filterValue, setFilterValue] = React.useState(initialFilterValue);
const handleFilterChange = (newFilterValue: any) => {
setFilterValue(newFilterValue);
console.log('New filter value:', newFilterValue);
};
return (
<FilterControl
fields={fields}
filterValue={filterValue}
onFilterValueChanged={handleFilterChange}
/>
);
};
export default App;
In this example, we define two fields: ‘name’ and ‘age’, each with a specific operator. The FilterControl
component is then rendered with these fields and an initial empty filter value.
The handleFilterChange
function updates the filter value state and logs the new value to the console, allowing you to see how the filter structure changes as users interact with it.
Advanced Filtering Techniques
Now that we’ve covered the basics, let’s explore some more advanced features of React Filter Control.
Grouping for Complex Queries
React Filter Control allows you to create nested filter groups, enabling complex logical combinations of filter criteria.
const complexFilterValue = {
groupName: 'and',
items: [
{
groupName: 'or',
items: [
{ field: 'name', operator: 'contains', value: 'John' },
{ field: 'name', operator: 'contains', value: 'Jane' }
]
},
{ field: 'age', operator: 'gte', value: 30 }
]
};
This filter structure would find all records where the name contains either “John” or “Jane”, AND the age is greater than or equal to 30.
Custom Operators for Precise Filtering
You can define custom operators to match your specific filtering needs:
const fields = [
{
name: 'status',
caption: 'Status',
operators: [
{ name: 'is', caption: 'Is' },
{ name: 'isNot', caption: 'Is Not' },
{ name: 'in', caption: 'In' }
]
}
];
This example adds multiple operators for a ‘status’ field, allowing users to create more nuanced filters.
Enhancing User Experience
React Filter Control is not just about functionality; it’s also about providing a smooth user experience.
Dynamic Field Updates
You can dynamically update the available fields based on user actions or data changes:
const [availableFields, setAvailableFields] = React.useState(initialFields);
// Later in your component...
useEffect(() => {
// Update fields based on some condition
if (someCondition) {
setAvailableFields([...initialFields, newField]);
}
}, [someCondition]);
return (
<FilterControl
fields={availableFields}
// ... other props
/>
);
This approach allows you to add or remove fields from the filter control dynamically, adapting to changing data or user permissions.
Styling and Customization
While React Filter Control provides a functional UI out of the box, you might want to customize its appearance to match your application’s design. You can achieve this by wrapping the FilterControl
component and applying your own CSS:
const StyledFilterControl = styled(FilterControl)`
.filter-group {
background-color: #f0f0f0;
border-radius: 4px;
padding: 8px;
}
.filter-item {
margin-bottom: 8px;
}
`;
This example uses styled-components to add custom styling to the filter groups and items.
Wrapping Up
React Filter Control offers a powerful solution for implementing complex filtering capabilities in React applications. By leveraging its features, you can create intuitive and flexible filtering interfaces that enhance data exploration and analysis for your users.
Remember to consult the official documentation for the most up-to-date API references and advanced usage scenarios. With React Filter Control, you’re well-equipped to tackle even the most demanding filtering requirements in your React projects.