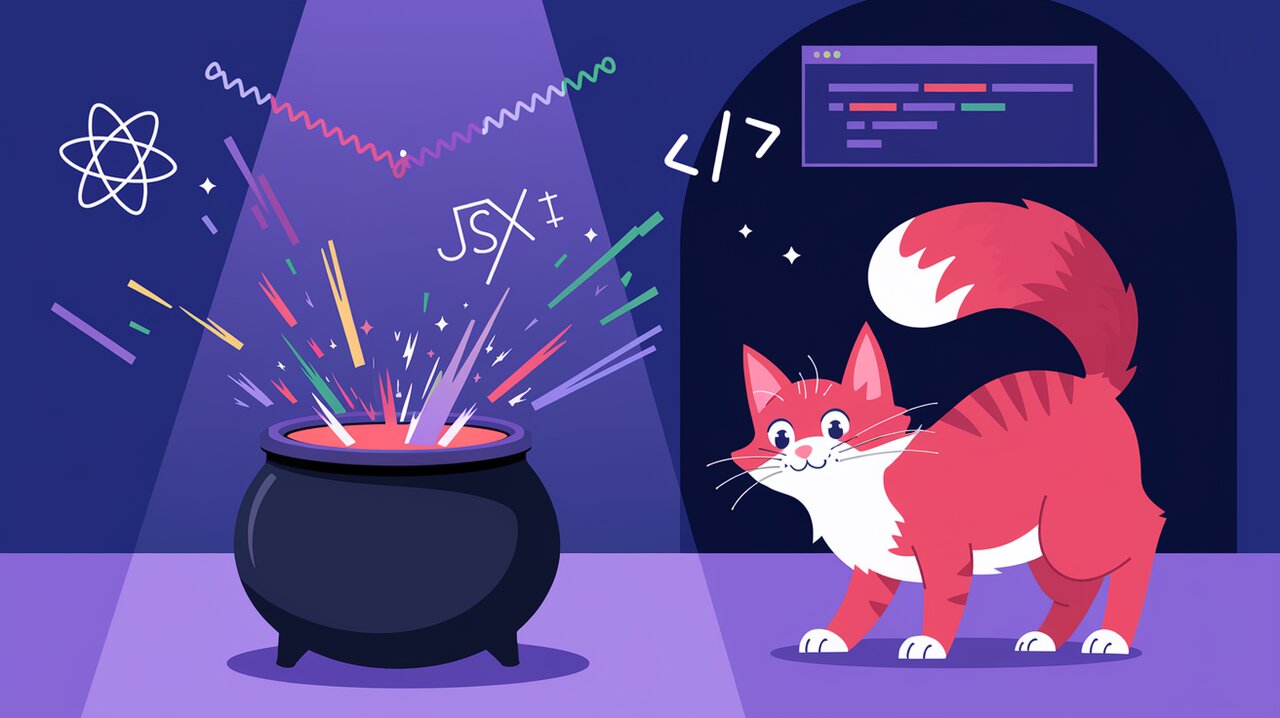
Unleashing JSX Magic: React-JSX-Parser's Dynamic Rendering Prowess
React-JSX-Parser is a powerful library that brings a new level of flexibility to React applications by allowing developers to parse and render JSX strings at runtime. This capability opens up a world of possibilities for creating dynamic and adaptable user interfaces. Whether you’re working with server-rendered content, building a content management system, or simply need to inject dynamic JSX into your React components, react-jsx-parser has got you covered.
Features
React-JSX-Parser comes packed with a set of features that make it a versatile tool for React developers:
- Dynamic JSX Parsing: Convert JSX strings into fully functional React components on the fly.
- Component Injection: Easily map custom components to JSX tags for seamless integration.
- Binding Support: Handle various types of property bindings, including expressions and named values.
- Security Measures: Built-in safeguards to prevent XSS attacks and other security vulnerabilities.
- Customizable Rendering: Fine-tune the parsing process with options for handling unknown elements and attributes.
Installation
Getting started with react-jsx-parser
is straightforward. You can install it using npm or yarn:
# Using npm
npm install react-jsx-parser
# Using yarn
yarn add react-jsx-parser
Basic Usage
Let’s dive into the basics of using react-jsx-parser in your React application.
Parsing Simple JSX Strings
To parse a simple JSX string, you can use the JsxParser
component like this:
import React from 'react';
import JsxParser from 'react-jsx-parser';
const App: React.FC = () => {
return (
<JsxParser
jsx={`
<div>
<h1>Hello, World!</h1>
<p>This is a dynamically rendered paragraph.</p>
</div>
`}
/>
);
};
In this example, the JSX string is parsed and rendered as if it were regular JSX code written directly in your component.
Injecting Custom Components
One of the most powerful features of react-jsx-parser is the ability to inject custom components into the parsed JSX:
import React from 'react';
import JsxParser from 'react-jsx-parser';
import CustomButton from './CustomButton';
const App: React.FC = () => {
return (
<JsxParser
components={{ CustomButton }}
jsx={`
<div>
<h1>Welcome to My App</h1>
<CustomButton label="Click me!" />
</div>
`}
/>
);
};
By passing your custom components through the components
prop, you can use them within your JSX strings just like any other React component.
Advanced Usage
Now that we’ve covered the basics, let’s explore some more advanced use cases for react-jsx-parser
.
Handling Dynamic Data
React-JSX-Parser allows you to work with dynamic data by using expression bindings:
import React from 'react';
import JsxParser from 'react-jsx-parser';
const App: React.FC = () => {
const user = { name: 'John Doe', age: 30 };
return (
<JsxParser
bindings={{ user }}
jsx={`
<div>
<h2>User Profile</h2>
<p>Name: {user.name}</p>
<p>Age: {user.age}</p>
</div>
`}
/>
);
};
By passing the user
object through the bindings
prop, we can access its properties within our JSX string.
Handling Events
While react-jsx-parser
doesn’t support inline function declarations for security reasons, you can still handle events by passing named event handlers:
import React from 'react';
import JsxParser from 'react-jsx-parser';
const App: React.FC = () => {
const handleClick = () => {
alert('Button clicked!');
};
return (
<JsxParser
bindings={{ handleClick }}
jsx={`
<button onClick={handleClick}>
Click me!
</button>
`}
/>
);
};
Customizing Parser Behavior
React-JSX-Parser provides several options to customize its behavior. Here’s an example that demonstrates some of these options:
import React from 'react';
import JsxParser from 'react-jsx-parser';
const App: React.FC = () => {
const handleError = (error: Error) => {
console.error('JSX Parsing Error:', error);
};
return (
<JsxParser
allowUnknownElements={false}
blacklistedAttrs={[/^on.+/i, 'className']}
onError={handleError}
jsx={`
<div className="container">
<h1>Custom Parsing</h1>
<UnknownComponent />
</div>
`}
/>
);
};
In this example, we’ve disabled unknown elements, blacklisted certain attributes, and provided an error handler for parsing issues.
Conclusion
React-JSX-Parser is a powerful tool that brings dynamic JSX rendering capabilities to your React applications. By allowing you to parse and render JSX strings at runtime, it opens up new possibilities for creating flexible and adaptable user interfaces. Whether you’re working with server-rendered content, building a CMS, or simply need more dynamic rendering options, react-jsx-parser provides the functionality you need.
As with any tool that parses and executes dynamic content, it’s important to use react-jsx-parser
responsibly and be aware of potential security implications. Always sanitize and validate any user-provided JSX to prevent XSS attacks and other vulnerabilities.
By mastering react-jsx-parser
, you’ll add a valuable skill to your React development toolkit, enabling you to create more dynamic and flexible applications with ease.