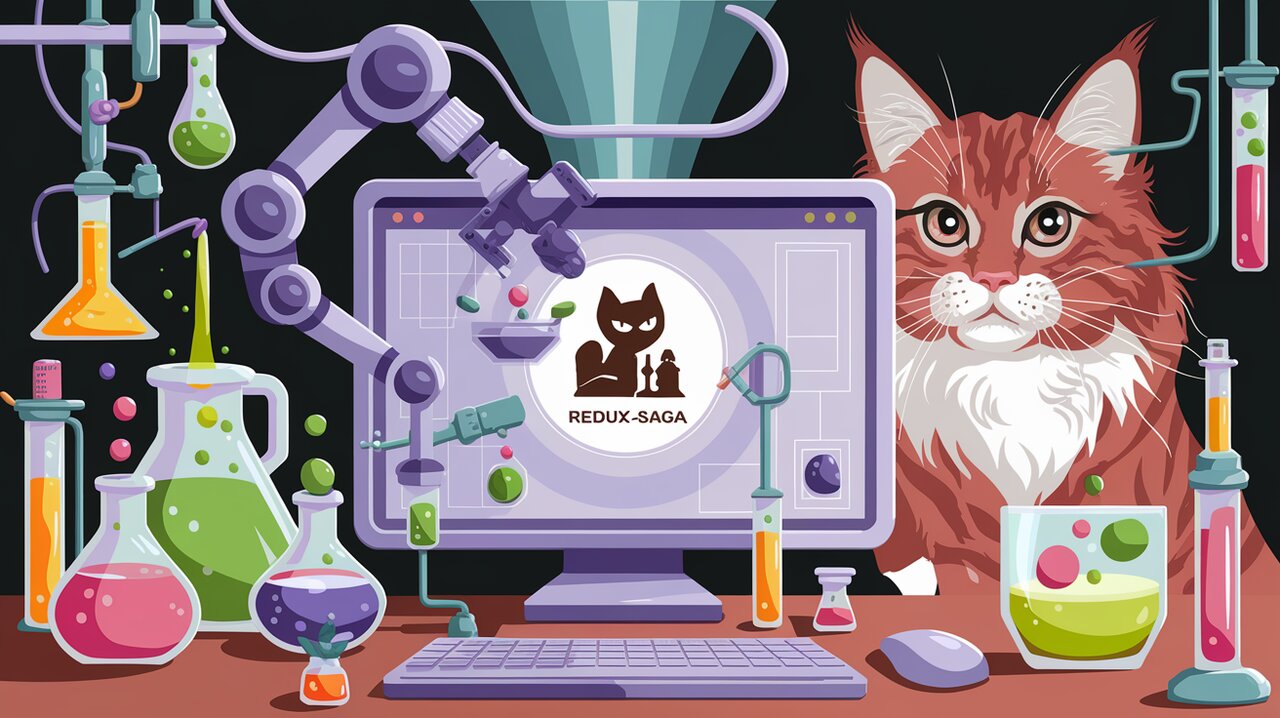
Unleashing the Power of Redux-Saga: Taming Asynchronous Beasts in React Applications
Unraveling the Redux-Saga Mystery
*Redux Saga emerges as a powerful ally in the React ecosystem, offering a sophisticated approach to managing side effects in applications. At its core, *Redux Saga leverages ES6 generator functions to create ‘sagas’ - isolated threads in your application dedicated to handling side effects. These sagas can be started, paused, and cancelled with ease, providing unprecedented control over asynchronous flows.
Key Features That Set Redux-Saga Apart
*Redux Saga boasts an impressive array of features that make it a go-to choice for developers tackling complex state management:
- Declarative Effects: Write asynchronous code that looks synchronous, enhancing readability and maintainability.
- Powerful Composition: Combine multiple sagas to handle complex async flows with ease.
- Side Effect Isolation: Keep your Redux actions pure by moving side effects into separate sagas.
- Efficient Execution: Utilize powerful effects like
takeLatest
to prevent race conditions. - Easy Testing: Test your sagas with a high degree of confidence, thanks to *Redux Saga’s predictable behavior.
Getting Started with Redux-Saga
To embark on your *Redux Saga journey, you’ll first need to install the library. Open your terminal and run:
npm install redux-saga
Or if you prefer yarn:
yarn add redux-saga
Setting Up Redux-Saga in Your Project
Once installed, integrating Redux Saga into your React application is straightforward. Let’s walk through the basic setup:
Configuring the Store
First, we need to create and configure the Redux store with the Redux Saga middleware:
import { createStore, applyMiddleware } from 'redux';
import createSagaMiddleware from 'redux-saga';
import rootReducer from './reducers';
import rootSaga from './sagas';
const sagaMiddleware = createSagaMiddleware();
const store = createStore(
rootReducer,
applyMiddleware(sagaMiddleware)
);
sagaMiddleware.run(rootSaga);
export default store;
In this setup, we create the saga middleware, apply it to the store, and then run our root saga. The rootSaga
is where we’ll define all our application’s sagas.
Creating Your First Saga
Let’s create a simple saga that responds to a user fetch action:
import { call, put, takeEvery } from 'redux-saga/effects';
import { fetchUserApi } from './api';
function* fetchUser(action) {
try {
const user = yield call(fetchUserApi, action.payload.userId);
yield put({ type: 'USER_FETCH_SUCCEEDED', user: user });
} catch (e) {
yield put({ type: 'USER_FETCH_FAILED', message: e.message });
}
}
function* userSaga() {
yield takeEvery('USER_FETCH_REQUESTED', fetchUser);
}
export default userSaga;
This saga watches for USER_FETCH_REQUESTED
actions and triggers the fetchUser
generator function when one occurs. The fetchUser
function makes an API call and dispatches a success or failure action based on the result.
Diving Deeper: Advanced Redux-Saga Techniques
As you become more comfortable with Redux Saga, you’ll want to explore its more advanced features. Let’s look at some powerful techniques:
Handling Concurrent Requests
Redux Saga provides several effect creators to manage concurrent operations. For instance, takeLatest
is particularly useful when you want to ensure only the result of the most recent request is processed:
import { takeLatest, call, put } from 'redux-saga/effects';
function* fetchLatestUser() {
try {
const user = yield call(fetchUserApi);
yield put({ type: 'LATEST_USER_FETCH_SUCCEEDED', user });
} catch (error) {
yield put({ type: 'LATEST_USER_FETCH_FAILED', error });
}
}
function* watchFetchLatestUser() {
yield takeLatest('FETCH_LATEST_USER', fetchLatestUser);
}
This saga will automatically cancel any ongoing fetchLatestUser
tasks when a new FETCH_LATEST_USER
action is dispatched, ensuring you’re always working with the most up-to-date data.
Composing Sagas
One of Redux Saga’s strengths is its ability to compose complex flows from simpler sagas. Here’s an example of how you might compose sagas for a user authentication flow:
import { all, call, put, take } from 'redux-saga/effects';
function* loginFlow() {
while (true) {
yield take('LOGIN_REQUEST');
const token = yield call(authorize);
if (token) {
yield put({ type: 'LOGIN_SUCCESS', token });
yield call(loadUserData);
yield take('LOGOUT');
yield call(clearSession);
}
}
}
function* rootSaga() {
yield all([
loginFlow(),
// other sagas...
]);
}
This composition creates a continuous loop that handles the entire login/logout cycle, including loading user data upon successful login.
Testing Sagas: Ensuring Reliability
One of the most significant advantages of Redux Saga is its testability. Let’s look at how we can test the fetchUser
saga we created earlier:
import { call, put } from 'redux-saga/effects';
import { fetchUser } from './sagas';
import { fetchUserApi } from './api';
describe('fetchUser Saga', () => {
const genObject = fetchUser({ payload: { userId: '123' } });
it('should call api and dispatch success action', () => {
const mockUser = { id: '123', name: 'John Doe' };
expect(genObject.next().value)
.toEqual(call(fetchUserApi, '123'));
expect(genObject.next(mockUser).value)
.toEqual(put({ type: 'USER_FETCH_SUCCEEDED', user: mockUser }));
expect(genObject.next().done).toBeTruthy();
});
it('should handle errors', () => {
const error = new Error('User not found');
expect(genObject.next().value)
.toEqual(call(fetchUserApi, '123'));
expect(genObject.throw(error).value)
.toEqual(put({ type: 'USER_FETCH_FAILED', message: error.message }));
expect(genObject.next().done).toBeTruthy();
});
});
This test suite verifies that our saga correctly calls the API and dispatches the appropriate actions based on the result. By testing each step of the generator, we can ensure our saga behaves correctly under various scenarios.
Conclusion: Harnessing the Full Potential of Redux-Saga
Redux Saga offers a powerful and flexible approach to managing side effects in React applications. By leveraging generator functions and a rich set of effects, it allows developers to write complex asynchronous logic that is both easy to understand and test. From basic API calls to intricate user flows, Redux Saga provides the tools needed to keep your application’s state management clean, predictable, and efficient.
As you continue to explore Redux Saga, you’ll discover even more ways to optimize your application’s performance and maintainability. Whether you’re building a small project or a large-scale application, Redux Saga’s ability to handle complex asynchronous operations makes it an invaluable tool in any React developer’s arsenal.
Remember, the key to mastering Redux Saga lies in practice and experimentation. Start small, gradually incorporate more advanced techniques, and soon you’ll be orchestrating complex side effects with ease, bringing a new level of robustness to your React applications.