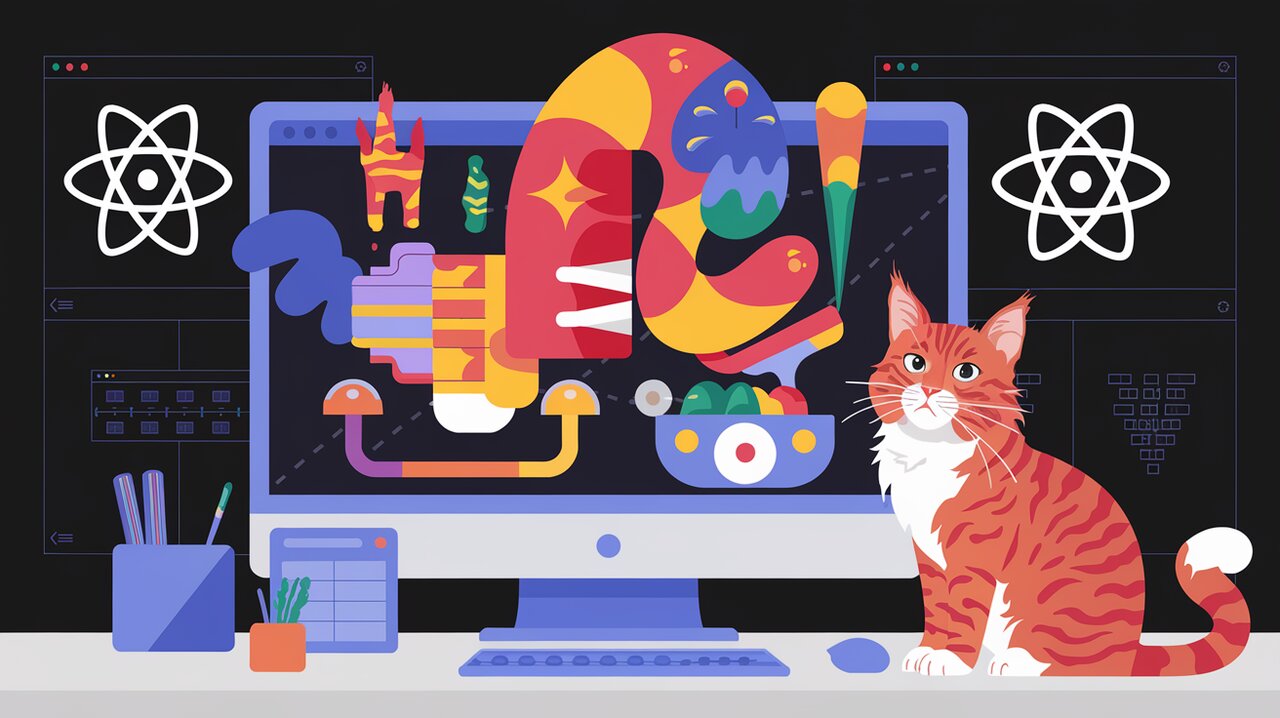
Revolutionizing SVG Integration in React
React SVG is a game-changing library that empowers developers to seamlessly inject SVG files into the DOM within React applications. This powerful tool bridges the gap between static SVG files and dynamic React components, offering a plethora of benefits for modern web development.
Key Features of React SVG
React SVG comes packed with features that make SVG manipulation a breeze:
- Asynchronous Loading: SVGs are loaded asynchronously, ensuring optimal performance.
- Caching: Loaded SVGs are cached, reducing redundant server requests.
- Customization: Extensive API for pre and post-injection manipulation of SVGs.
- Accessibility: Built-in support for adding ARIA attributes.
- Error Handling: Robust error management with fallback options.
- Script Evaluation: Optional execution of script blocks within SVGs.
Getting Started with React SVG
Installation
To start using React SVG in your project, you can install it via npm or yarn:
npm install react-svg
# or
yarn add react-svg
Basic Usage
Let’s dive into a simple example to demonstrate how easy it is to use React SVG:
import { createRoot } from 'react-dom/client';
import { ReactSVG } from 'react-svg';
const App = () => (
<ReactSVG src="path/to/your/svg.svg" />
);
const container = document.getElementById('root');
const root = createRoot(container);
root.render(<App />);
In this basic setup, React SVG will load the SVG file from the specified src
and inject it into the DOM. The component automatically handles the asynchronous loading and error states.
Advanced Techniques
Customizing SVG Before Injection
React SVG allows you to manipulate the SVG element before it’s injected into the DOM:
import { ReactSVG } from 'react-svg';
const EnhancedSVG = () => (
<ReactSVG
src="logo.svg"
beforeInjection={(svg) => {
svg.classList.add('logo-svg');
svg.setAttribute('style', 'width: 200px');
}}
/>
);
This example adds a class and sets a width to the SVG element before it’s injected.
Handling Post-Injection Actions
You can also perform actions after the SVG has been injected:
import { ReactSVG } from 'react-svg';
const AnimatedSVG = () => (
<ReactSVG
src="animation.svg"
afterInjection={(svg) => {
svg.querySelector('#animate-me').beginElement();
}}
/>
);
Here, we trigger an animation within the SVG after it has been injected into the DOM.
Error Handling and Fallbacks
React SVG provides robust error handling capabilities:
import { ReactSVG } from 'react-svg';
const SafeSVG = () => (
<ReactSVG
src="potentially-broken.svg"
fallback={() => <span>Error loading SVG!</span>}
onError={(error) => console.error(error)}
/>
);
This setup displays a fallback component if the SVG fails to load and logs the error to the console.
Optimizing Performance
Caching and Request Optimization
React SVG employs smart caching to enhance performance:
import { ReactSVG } from 'react-svg';
const OptimizedSVG = () => (
<ReactSVG
src="heavy-svg.svg"
useRequestCache={true}
httpRequestWithCredentials={false}
/>
);
By enabling useRequestCache
, React SVG ensures that multiple instances of the same SVG only require a single server request.
Controlling Script Execution
For SVGs containing scripts, you can control their execution:
import { ReactSVG } from 'react-svg';
const ScriptedSVG = () => (
<ReactSVG
src="interactive.svg"
evalScripts="always"
/>
);
The evalScripts
prop allows you to specify when and if scripts within the SVG should be executed.
Enhancing Accessibility
React SVG makes it easy to improve the accessibility of your SVGs:
import { ReactSVG } from 'react-svg';
const AccessibleSVG = () => (
<ReactSVG
src="icon.svg"
title="User Profile Icon"
desc="A stylized silhouette representing a user profile"
/>
);
This example adds a title and description to the SVG, enhancing its accessibility for screen readers.
Conclusion: Elevating SVG Integration in React
React SVG stands out as an indispensable tool for developers looking to seamlessly integrate and manipulate SVGs within React applications. Its robust feature set, from asynchronous loading and caching to extensive customization options, makes it a powerful ally in creating dynamic and efficient user interfaces. By simplifying the process of SVG injection and manipulation, React SVG enables developers to focus on crafting engaging visual experiences without getting bogged down in the intricacies of SVG handling. Whether you’re building complex data visualizations or simply enhancing your UI with scalable graphics, React SVG provides the flexibility and performance needed to bring your creative visions to life in the React ecosystem.