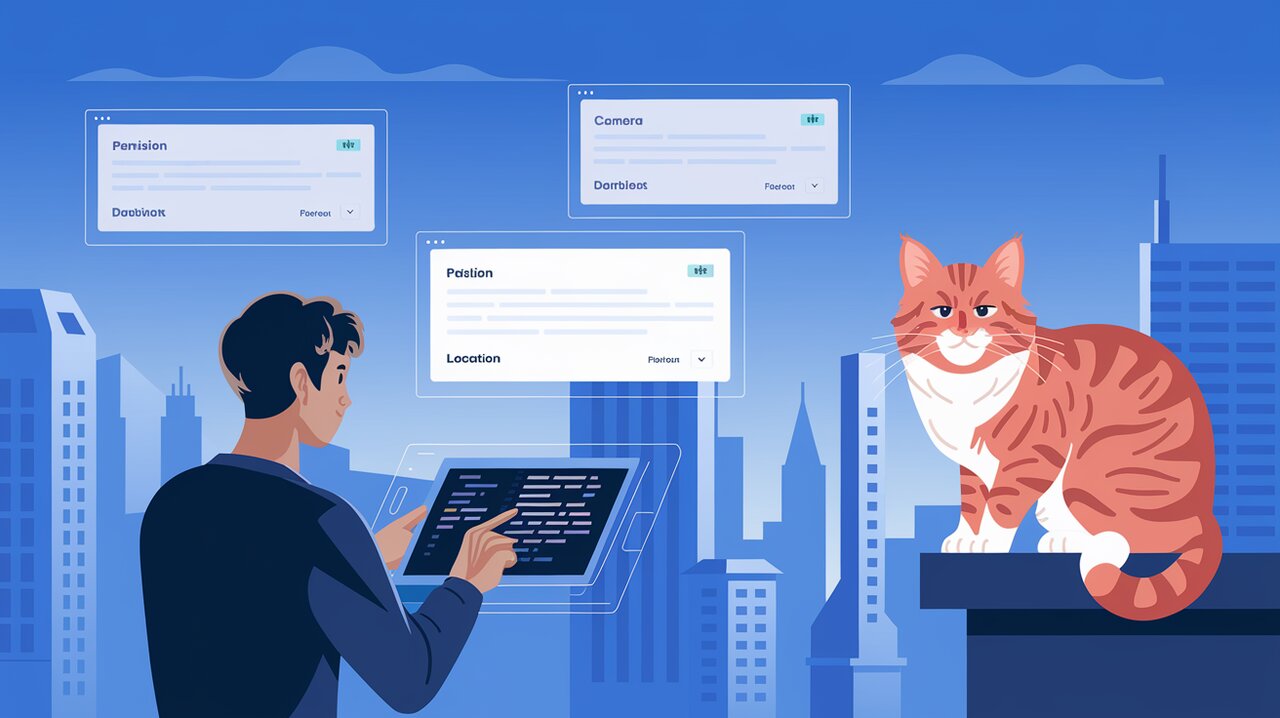
Unlocking Your App's Potential with react-native-permissions
In the ever-evolving landscape of mobile app development, managing user permissions is crucial for both functionality and user privacy. react-native-permissions emerges as a powerful solution for React Native developers, offering a unified API to handle permissions across iOS, Android, and Windows platforms. Let’s dive into how this library can streamline your permission management process and enhance your app’s user experience.
Empowering Your App with Permissions
react-native-permissions provides a comprehensive set of tools to check, request, and manage various device permissions. From accessing the camera to reading contacts, this library simplifies the complex world of platform-specific permission handling.
Key Features
- Cross-Platform Compatibility: Works seamlessly on iOS, Android, and Windows.
- Unified API: Consistent method calls across all supported platforms.
- Granular Control: Fine-tuned permission management for specific features.
- Customizable Rationale: Explain permission requests to users on Android.
- Notification Handling: Special methods for dealing with notification permissions.
Getting Started
To begin using react-native-permissions in your project, you’ll need to install the package and configure it for each platform you’re targeting.
Installation
First, add the library to your project:
npm install react-native-permissions
# or
yarn add react-native-permissions
Platform-Specific Setup
iOS Configuration
For iOS, you’ll need to modify your Podfile
to specify which permissions your app requires:
require_relative '../node_modules/react-native/scripts/react_native_pods'
require_relative '../node_modules/react-native-permissions/scripts/setup'
# ...
target 'YourApp' do
# ...
setup_permissions([
'Camera',
'PhotoLibrary',
'Microphone',
# Add other permissions as needed
])
end
After modifying the Podfile
, run pod install
in your iOS directory.
Next, update your Info.plist
file with usage descriptions for each permission:
<key>NSCameraUsageDescription</key>
<string>We need access to your camera for taking profile pictures.</string>
<key>NSPhotoLibraryUsageDescription</key>
<string>We need access to your photo library for selecting profile pictures.</string>
<key>NSMicrophoneUsageDescription</key>
<string>We need access to your microphone for voice messages.</string>
Android Configuration
For Android, add the required permissions to your AndroidManifest.xml
:
<manifest xmlns:android="http://schemas.android.com/apk/res/android">
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<!-- Add other permissions as needed -->
</manifest>
Windows Configuration
For Windows, open your project solution file and modify the Package.appxmanifest
to include the capabilities your app needs.
Implementing Permissions in Your App
Now that we’ve set up react-native-permissions, let’s explore how to use it in your React Native application.
Checking Permission Status
Before requesting a permission, it’s good practice to check its current status:
import { check, PERMISSIONS, RESULTS } from 'react-native-permissions';
const checkCameraPermission = async () => {
const result = await check(PERMISSIONS.IOS.CAMERA);
switch (result) {
case RESULTS.UNAVAILABLE:
console.log('This feature is not available on this device');
break;
case RESULTS.DENIED:
console.log('Permission has not been requested / is denied but requestable');
break;
case RESULTS.GRANTED:
console.log('The permission is granted');
break;
case RESULTS.BLOCKED:
console.log('The permission is denied and not requestable anymore');
break;
}
};
Requesting Permissions
When you need to request a permission:
import { request, PERMISSIONS } from 'react-native-permissions';
const requestCameraPermission = async () => {
const result = await request(PERMISSIONS.IOS.CAMERA);
if (result === RESULTS.GRANTED) {
console.log('Camera permission granted');
// Proceed with using the camera
}
};
Handling Multiple Permissions
For apps that require multiple permissions, you can use the requestMultiple
function:
import { requestMultiple, PERMISSIONS } from 'react-native-permissions';
const requestMultiplePermissions = async () => {
const statuses = await requestMultiple([
PERMISSIONS.IOS.CAMERA,
PERMISSIONS.IOS.PHOTO_LIBRARY,
PERMISSIONS.IOS.MICROPHONE,
]);
console.log('Camera', statuses[PERMISSIONS.IOS.CAMERA]);
console.log('Photo Library', statuses[PERMISSIONS.IOS.PHOTO_LIBRARY]);
console.log('Microphone', statuses[PERMISSIONS.IOS.MICROPHONE]);
};
Advanced Usage
Customizing Android Rationale
On Android, you can provide a custom rationale when requesting permissions:
import { request, PERMISSIONS } from 'react-native-permissions';
const requestWithRationale = async () => {
const result = await request(PERMISSIONS.ANDROID.CAMERA, {
title: 'Camera Permission',
message: 'We need access to your camera to take profile pictures.',
buttonPositive: 'OK',
buttonNegative: 'Cancel',
});
if (result === RESULTS.GRANTED) {
// Permission granted, proceed with camera usage
}
};
Handling Notifications
Notification permissions require special handling:
import { checkNotifications, requestNotifications } from 'react-native-permissions';
const handleNotificationPermission = async () => {
const { status } = await checkNotifications();
if (status !== 'granted') {
const { status: newStatus } = await requestNotifications(['alert', 'sound']);
console.log('Notification permission status:', newStatus);
}
};
Opening Settings
If a permission is blocked, you might want to guide the user to the app settings:
import { openSettings } from 'react-native-permissions';
const openAppSettings = async () => {
await openSettings().catch(() => console.warn('Cannot open settings'));
};
Best Practices
-
Check Before Requesting: Always check the permission status before requesting it to provide a smoother user experience.
-
Explain the Need: Clearly communicate why your app needs each permission, both in the UI and in the
Info.plist
orAndroidManifest.xml
. -
Handle Denials Gracefully: Provide alternative functionality or clear instructions when permissions are denied.
-
Respect User Choices: If a user denies a permission, don’t repeatedly ask for it. Instead, explain the limitations and offer a way to grant the permission later.
-
Use Rationale on Android: Utilize the rationale feature on Android to explain permission requests clearly.
-
Test Thoroughly: Test your app’s behavior under all permission scenarios, including when permissions are granted, denied, or revoked.
Conclusion
react-native-permissions simplifies the complex task of managing permissions in React Native applications. By providing a unified API across iOS, Android, and Windows platforms, it allows developers to focus on creating great user experiences while ensuring proper handling of sensitive device features.
Remember, with great power comes great responsibility. Always respect user privacy and only request permissions that are essential for your app’s functionality. By following the best practices outlined in this guide and leveraging the capabilities of react-native-permissions, you can create robust, user-friendly apps that seamlessly integrate with device features while maintaining user trust and compliance with platform guidelines.
As you continue to develop your React Native applications, keep exploring the extensive features of react-native-permissions to stay on top of evolving platform requirements and user expectations. Happy coding!