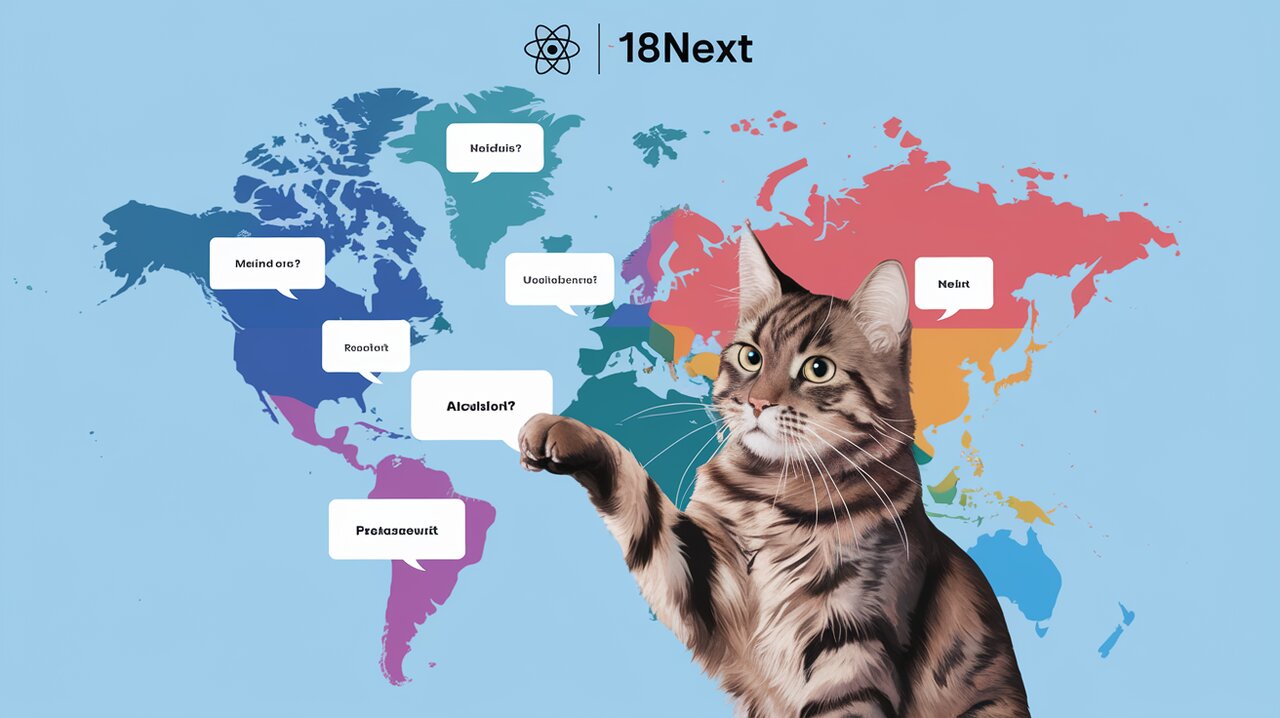
Unlocking Multilingual Magic: Mastering React-i18next for Global Audiences
React-i18next is a powerful internationalization framework for React applications, built on top of the popular i18next library. It provides a seamless way to add multilingual support to your React projects, allowing you to reach a global audience with ease. In this comprehensive guide, we’ll explore how to leverage react-i18next to create dynamic, multilingual applications that can adapt to users’ language preferences.
Features
React-i18next offers a rich set of features that make internationalization a breeze:
- Simple integration with React components
- Support for hooks in functional components
- Automatic language detection
- Easy loading of translation files
- Pluralization and interpolation support
- Nested translations
- Context-aware translations
- Server-side rendering compatibility
Installation
To get started with react-i18next, you’ll need to install both i18next and react-i18next packages. Open your terminal and run the following command:
npm install i18next react-i18next
For Yarn users:
yarn add i18next react-i18next
Basic Usage
Configuring i18next
The first step is to set up and configure i18next. Create a new file called i18n.js
in your project’s src
directory with the following content:
import i18n from 'i18next';
import { initReactI18next } from 'react-i18next';
i18n
.use(initReactI18next)
.init({
resources: {
en: {
translation: {
"welcome": "Welcome to React and react-i18next"
}
},
fr: {
translation: {
"welcome": "Bienvenue à React et react-i18next"
}
}
},
lng: "en",
fallbackLng: "en",
interpolation: {
escapeValue: false
}
});
export default i18n;
This configuration sets up English as the default language and includes a simple translation for a welcome message in both English and French.
Using Translations in Components
Now that we have our i18next instance configured, let’s use it in a React component. Here’s a simple example using the useTranslation
hook:
import React from 'react';
import { useTranslation } from 'react-i18next';
function Welcome() {
const { t } = useTranslation();
return <h1>{t('welcome')}</h1>;
}
export default Welcome;
In this example, the useTranslation
hook provides us with the t
function, which we use to translate our “welcome” key.
Changing Languages
To allow users to switch languages, you can create a language selector component:
import React from 'react';
import { useTranslation } from 'react-i18next';
function LanguageSelector() {
const { i18n } = useTranslation();
const changeLanguage = (lng: string) => {
i18n.changeLanguage(lng);
};
return (
<div>
<button onClick={() => changeLanguage('en')}>English</button>
<button onClick={() => changeLanguage('fr')}>Français</button>
</div>
);
}
export default LanguageSelector;
This component uses the i18n
object provided by useTranslation
to change the language when a button is clicked.
Advanced Usage
Interpolation
React-i18next supports variable interpolation in your translations. Here’s how you can use it:
// In your translation file
{
"greeting": "Hello, {{name}}!"
}
// In your React component
import React from 'react';
import { useTranslation } from 'react-i18next';
function Greeting({ name }) {
const { t } = useTranslation();
return <p>{t('greeting', { name })}</p>;
}
export default Greeting;
Pluralization
Handling plurals is easy with react-i18next. Here’s an example:
// In your translation file
{
"items": "{{count}} item",
"items_plural": "{{count}} items"
}
// In your React component
import React from 'react';
import { useTranslation } from 'react-i18next';
function ItemCount({ count }) {
const { t } = useTranslation();
return <p>{t('items', { count })}</p>;
}
export default ItemCount;
Using the Trans Component
For more complex translations that include HTML or nested React components, you can use the Trans
component:
import React from 'react';
import { Trans } from 'react-i18next';
function MyComponent() {
return (
<Trans i18nKey="myKey">
This is <strong>bold</strong> and <i>italic</i> text.
</Trans>
);
}
export default MyComponent;
Loading Translations from a Server
In real-world applications, you’ll often want to load translations from a server. You can use the i18next-http-backend
plugin for this:
import i18n from 'i18next';
import { initReactI18next } from 'react-i18next';
import Backend from 'i18next-http-backend';
i18n
.use(Backend)
.use(initReactI18next)
.init({
fallbackLng: 'en',
backend: {
loadPath: '/locales/{{lng}}/{{ns}}.json',
},
});
export default i18n;
This setup will load translation files from your server based on the specified path.
Conclusion
React-i18next provides a powerful and flexible solution for internationalizing React applications. By following the steps outlined in this guide, you can create multilingual applications that cater to a global audience. From basic translations to advanced features like interpolation, pluralization, and server-side loading, react-i18next
offers everything you need to make your app speak multiple languages fluently.
Remember that internationalization is an ongoing process. As your application grows, you’ll need to continuously update and manage your translations. Consider using translation management tools and involving professional translators to ensure the quality and accuracy of your localized content.
With react-i18next
, you’re well-equipped to create React applications that can truly connect with users around the world, breaking down language barriers and expanding your app’s reach. Happy internationalizing!