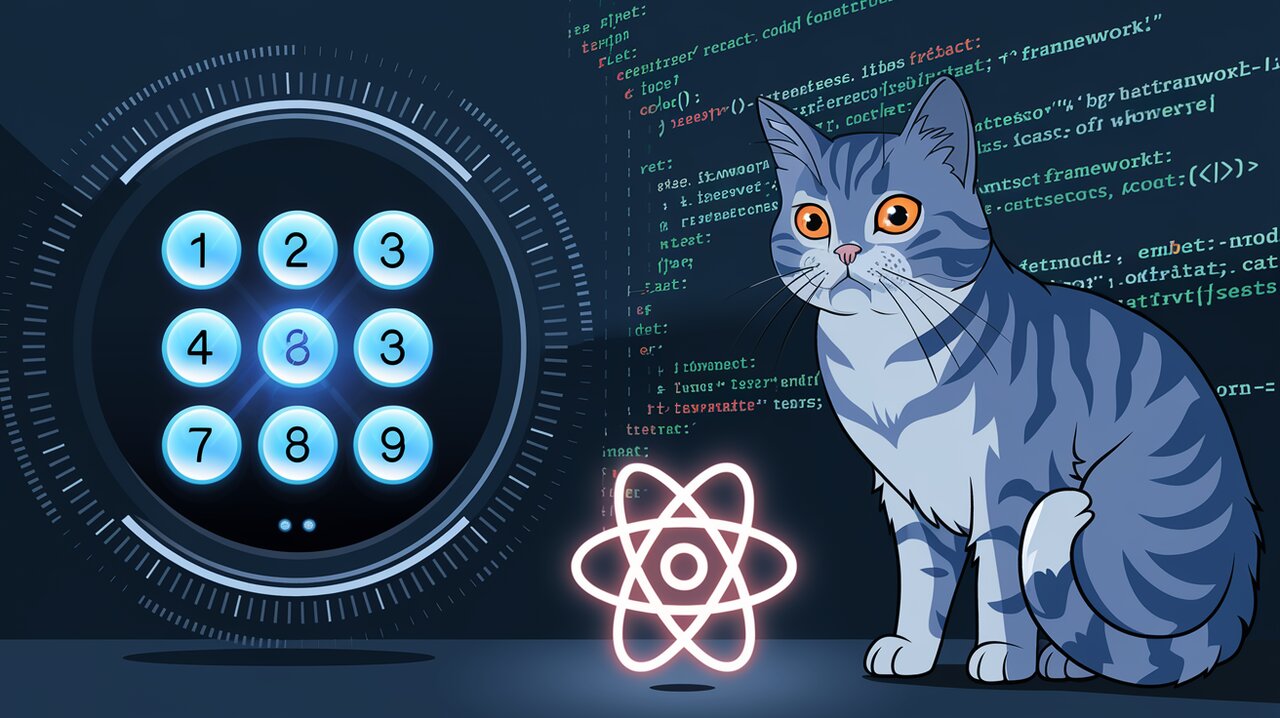
React Code Input is a powerful and flexible component that simplifies the process of creating PIN code entry fields in React applications. Whether you’re building a secure login system, a verification process, or any feature requiring code input, this library provides an elegant solution with customizable options to fit your specific needs.
Unlocking the Power of React Code Input
React Code Input offers a range of features that make it an excellent choice for developers looking to implement code entry functionality:
- Support for various input types (number, text, password)
- Customizable number of input fields
- Easy styling options
- Validation capabilities
- Accessibility features
Let’s dive into how you can harness these features in your React projects.
Installing the Secret Key
To begin your journey with React Code Input, you’ll need to install the library. Open your terminal and run one of the following commands:
npm install react-code-input
or if you prefer yarn:
yarn add react-code-input
Basic Usage: Cracking the Code
Once installed, you can start using React Code Input in your components. Here’s a simple example to get you started:
import React from 'react';
import ReactCodeInput from 'react-code-input';
const PINEntry: React.FC = () => {
return (
<ReactCodeInput
type='number'
fields={6}
onChange={(value) => console.log(value)}
/>
);
};
export default PINEntry;
This code creates a numeric PIN input with 6 fields. The onChange
prop allows you to handle the input value as it’s entered.
Customizing Your Secret Code Chamber
React Code Input offers extensive customization options to match your application’s design. Let’s explore some advanced usage scenarios:
Styling Your PIN Pad
You can easily style your input fields using the inputStyle
and inputStyleInvalid
props:
import React from 'react';
import ReactCodeInput from 'react-code-input';
const StyledPINEntry: React.FC = () => {
const inputStyle = {
fontFamily: 'monospace',
margin: '4px',
MozAppearance: 'textfield',
width: '15px',
borderRadius: '3px',
fontSize: '14px',
height: '26px',
paddingLeft: '7px',
backgroundColor: 'black',
color: 'lightskyblue',
border: '1px solid lightskyblue'
};
const inputStyleInvalid = {
...inputStyle,
color: 'red',
border: '1px solid red'
};
return (
<ReactCodeInput
type='number'
fields={6}
inputStyle={inputStyle}
inputStyleInvalid={inputStyleInvalid}
/>
);
};
export default StyledPINEntry;
This creates a sleek, cyberpunk-inspired PIN input that changes style when invalid.
Securing the Vault: Password Input
For sensitive information, you can use the password type:
import React from 'react';
import ReactCodeInput from 'react-code-input';
const SecurePINEntry: React.FC = () => {
return (
<ReactCodeInput
type='password'
fields={4}
placeholder='●'
/>
);
};
export default SecurePINEntry;
This creates a 4-digit password input where entered characters are masked for security.
Advanced Features: Booby Traps and Secret Passages
React Code Input comes with several advanced features to enhance your code entry experience:
Validation: Defusing the Bomb
You can implement custom validation logic using the isValid
prop:
import React, { useState } from 'react';
import ReactCodeInput from 'react-code-input';
const ValidatedPINEntry: React.FC = () => {
const [isValid, setIsValid] = useState(true);
const handleChange = (value: string) => {
setIsValid(value.length === 6 && parseInt(value) % 2 === 0);
};
return (
<ReactCodeInput
type='number'
fields={6}
onChange={handleChange}
isValid={isValid}
/>
);
};
export default ValidatedPINEntry;
This example validates that the entered PIN is 6 digits long and an even number.
Autocompletion: The Master Key
For improved user experience, you can enable autocompletion:
import React from 'react';
import ReactCodeInput from 'react-code-input';
const AutocompletePINEntry: React.FC = () => {
return (
<ReactCodeInput
type='number'
fields={6}
autoComplete='one-time-code'
/>
);
};
export default AutocompletePINEntry;
This allows browsers to suggest previously used codes, which can be helpful for returning users.
Conclusion: The Code is Cracked
React Code Input provides a robust solution for implementing PIN and code entry fields in your React applications. Its flexibility, ease of use, and extensive customization options make it an excellent choice for developers looking to enhance their user authentication and verification processes.
By leveraging the power of React Code Input, you can create secure, user-friendly, and visually appealing code entry experiences that will keep your users coming back for more. Whether you’re building a banking app, a secure messaging platform, or any application requiring code input, this library has got you covered.
Remember, with great power comes great responsibility. Use React Code Input wisely, and may your codes always be secure and your users always satisfied!
For more React component magic, check out our articles on mastering React Datepicker and elevating your header game with React Headroom. Happy coding!