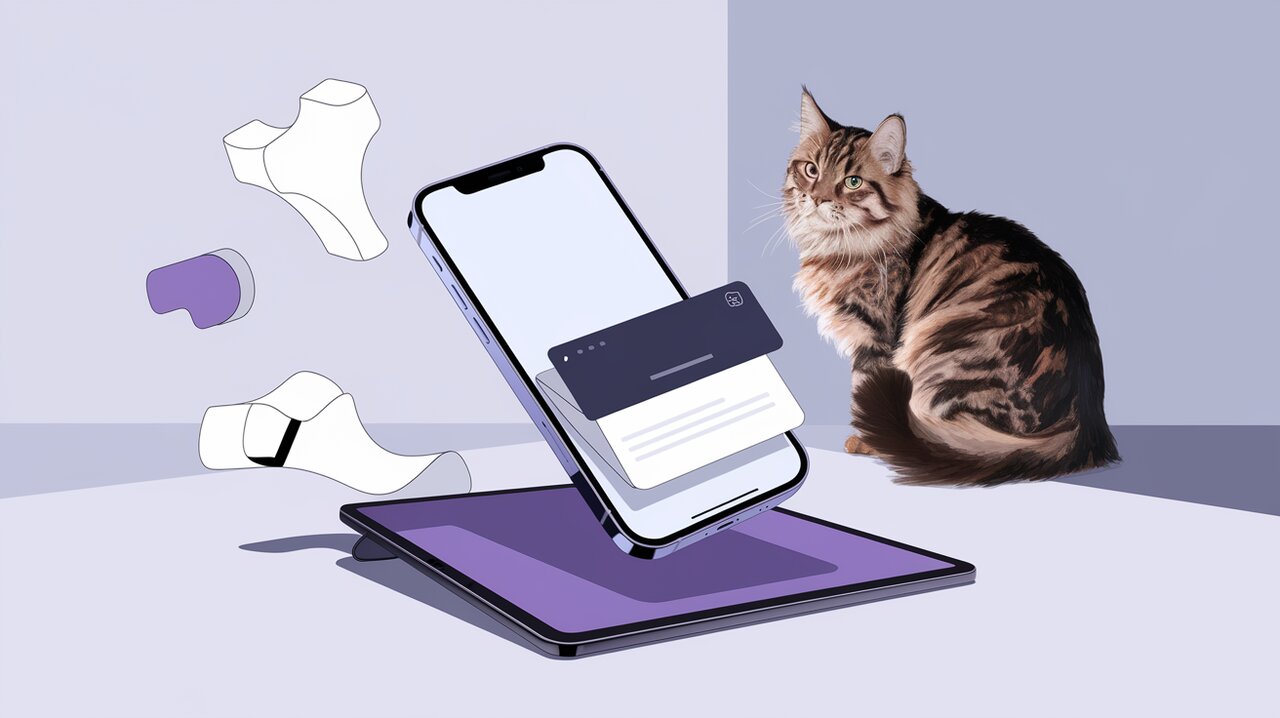
Unveiling Vaul: The Drawer Component That Will Slide Into Your React Heart
In the ever-evolving landscape of web development, creating seamless and intuitive user interfaces for mobile and tablet devices remains a top priority. Vaul emerges as a game-changing solution for React developers, offering a versatile and customizable drawer component that can revolutionize how we approach dialog and content presentation on smaller screens.
Unlocking the Power of Vaul
At its core, Vaul is an unstyled drawer component designed specifically for React applications. Its primary purpose is to serve as a replacement for traditional dialog components on tablet and mobile devices, providing a more natural and fluid user experience. By leveraging the power of drawers, Vaul allows developers to create interfaces that feel native to touch-based devices, enhancing user engagement and interaction.
Key Features That Set Vaul Apart
- Unstyled Architecture: Vaul comes with no predefined styles, giving developers complete control over the look and feel of their drawers.
- Responsive Design: Seamlessly adapts to various screen sizes, making it perfect for both mobile and tablet interfaces.
- Customizable Behavior: Offers fine-grained control over drawer animations, snap points, and interaction patterns.
- Accessibility: Built with accessibility in mind, ensuring that your interfaces remain usable for all users.
- TypeScript Support: Fully typed, providing excellent developer experience and code reliability.
Getting Started with Vaul
Installation
Integrating Vaul into your React project is a breeze. You can install it using npm or yarn:
npm install vaul
# or
yarn add vaul
Basic Implementation
Let’s dive into a simple example to showcase how easy it is to implement a drawer using Vaul:
import React from 'react';
import { Drawer } from 'vaul';
const SimpleDrawer: React.FC = () => {
return (
<Drawer.Root>
<Drawer.Trigger>Open Drawer</Drawer.Trigger>
<Drawer.Portal>
<Drawer.Content>
<Drawer.Title>Welcome to Vaul</Drawer.Title>
<p>This is a basic drawer implementation.</p>
</Drawer.Content>
<Drawer.Overlay />
</Drawer.Portal>
</Drawer.Root>
);
};
export default SimpleDrawer;
In this example, we’ve created a basic drawer with a trigger button, content, and an overlay. The Drawer.Root
component wraps everything, providing the context for the drawer’s functionality. The Drawer.Trigger
acts as the button to open the drawer, while Drawer.Content
houses the actual content of the drawer. The Drawer.Overlay
creates a backdrop when the drawer is open.
Understanding Drawer Components
Let’s break down the key components of Vaul:
- Drawer.Root: The main container that manages the state of the drawer.
- Drawer.Trigger: The element that opens the drawer when interacted with.
- Drawer.Portal: Renders the drawer content in a React portal, ensuring proper stacking context.
- Drawer.Content: The container for your drawer’s content.
- Drawer.Title: An optional, accessible title for your drawer.
- Drawer.Overlay: Creates a backdrop behind the open drawer.
Each of these components plays a crucial role in creating a fully functional and accessible drawer interface.
Advanced Vaul Techniques
Controlled Drawers
For more complex scenarios, you might want to control the drawer’s state programmatically. Vaul makes this easy with controlled components:
import React, { useState } from 'react';
import { Drawer } from 'vaul';
const ControlledDrawer: React.FC = () => {
const [isOpen, setIsOpen] = useState(false);
return (
<Drawer.Root open={isOpen} onOpenChange={setIsOpen}>
<button onClick={() => setIsOpen(true)}>Open Controlled Drawer</button>
<Drawer.Portal>
<Drawer.Content>
<h2>Controlled Drawer</h2>
<p>This drawer's state is managed by React state.</p>
<button onClick={() => setIsOpen(false)}>Close</button>
</Drawer.Content>
<Drawer.Overlay />
</Drawer.Portal>
</Drawer.Root>
);
};
export default ControlledDrawer;
In this example, we use React’s useState
hook to manage the drawer’s open state. The open
and onOpenChange
props on Drawer.Root
allow us to control the drawer programmatically.
Customizing Snap Points
Vaul allows you to define custom snap points, giving users more flexibility in how they interact with the drawer:
import React from 'react';
import { Drawer } from 'vaul';
const SnapPointDrawer: React.FC = () => {
return (
<Drawer.Root snapPoints={[0.5, 1]}>
<Drawer.Trigger>Open Snap Drawer</Drawer.Trigger>
<Drawer.Portal>
<Drawer.Content>
<p>Drag me to snap at 50% or 100% of the screen height!</p>
</Drawer.Content>
<Drawer.Overlay />
</Drawer.Portal>
</Drawer.Root>
);
};
export default SnapPointDrawer;
The snapPoints
prop defines the positions where the drawer will naturally snap to. In this case, it will snap to either 50% or 100% of the screen height.
Nested Drawers
For complex interfaces, Vaul supports nested drawers, allowing you to create multi-level navigation or content hierarchies:
import React from 'react';
import { Drawer } from 'vaul';
const NestedDrawers: React.FC = () => {
return (
<Drawer.Root>
<Drawer.Trigger>Open Parent Drawer</Drawer.Trigger>
<Drawer.Portal>
<Drawer.Content>
<h2>Parent Drawer</h2>
<Drawer.Root>
<Drawer.Trigger>Open Child Drawer</Drawer.Trigger>
<Drawer.Portal>
<Drawer.Content>
<h3>Child Drawer</h3>
<p>This is a nested drawer!</p>
</Drawer.Content>
<Drawer.Overlay />
</Drawer.Portal>
</Drawer.Root>
</Drawer.Content>
<Drawer.Overlay />
</Drawer.Portal>
</Drawer.Root>
);
};
export default NestedDrawers;
This example demonstrates how you can nest drawers within each other, creating a hierarchical structure for complex UIs.
Wrapping Up: The Vaul-ue Proposition
Vaul stands out as a powerful and flexible solution for implementing drawer interfaces in React applications. Its unstyled nature provides unlimited customization possibilities, while its thoughtful API ensures that developers can create accessible and responsive drawer components with ease.
By leveraging Vaul in your projects, you can significantly enhance the user experience on mobile and tablet devices, replacing traditional dialogs with smooth, intuitive drawer interactions. Whether you’re building a complex e-commerce platform, a sleek mobile app, or anything in between, Vaul offers the tools you need to create engaging and user-friendly interfaces.
As you continue to explore the capabilities of Vaul, you’ll discover even more ways to optimize your React applications for touch-based devices. So why wait? Dive into Vaul today and start sliding your way to better user interfaces!