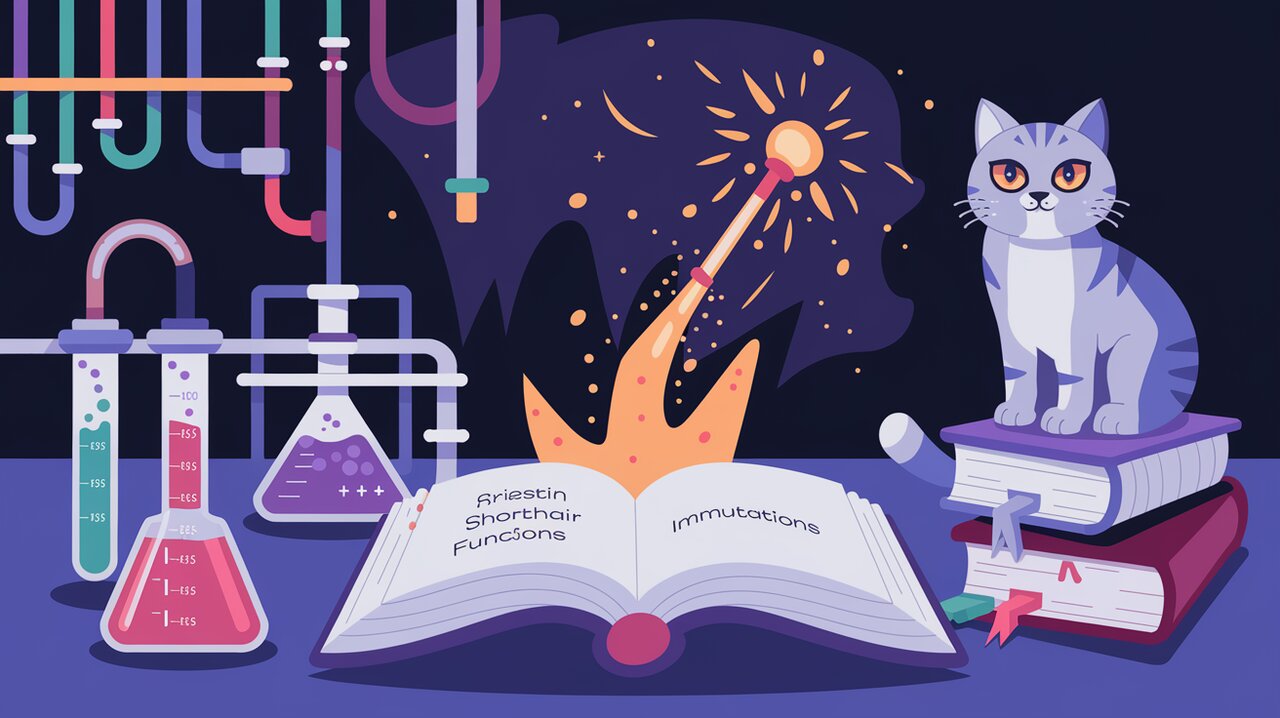
Updeep: The Magical Wand for Immutable Object Sorcery in React
In the enchanting realm of React development, managing state can often feel like navigating a labyrinth of nested objects and arrays. Enter updeep, a magical library that promises to transform the way you handle immutable updates in your applications. Like a skilled sorcerer’s wand, updeep allows you to declare your desired changes and effortlessly updates your state, all while maintaining the sanctity of immutability.
Unveiling the Magic of Updeep
updeep is not just another utility library; it’s a powerful tool designed to make working with immutable data structures in JavaScript a delightful experience. At its core, updeep allows you to update deeply nested objects and arrays in a declarative and immutable manner, making it an invaluable asset for React developers.
Key Enchantments
- Immutability by Default: Everything returned by updeep is frozen, ensuring true immutability.
- Declarative Updates: Specify what you want to change, and updeep takes care of the rest.
- Deep Updates: Effortlessly update nested properties without the need for deep cloning.
- Performance Optimized: Returns the same instance if no changes are made, perfect for reference equality checks.
- Flexible API: Works well with other functional programming libraries like lodash/fp or Ramda.
Summoning Updeep to Your Project
Before we dive into the arcane arts of immutable updates, let’s summon updeep to our project. Open your terminal and cast the following spell:
npm install updeep
# or if you prefer yarn
yarn add updeep
Basic Incantations
Let’s start with some basic spells to get a feel for updeep’s power.
The Fundamental Update Spell
At the heart of updeep is the u
function. It’s the primary incantation you’ll use to perform updates.
import u from 'updeep';
const person = {
name: { first: 'Harry', last: 'Potter' },
house: 'Gryffindor',
age: 11
};
const updatedPerson = u({
name: { first: 'Hermione' },
age: (age) => age + 1
}, person);
console.log(updatedPerson);
// Output: { name: { first: 'Hermione', last: 'Potter' }, house: 'Gryffindor', age: 12 }
In this example, we’ve changed the first name and incremented the age. Notice how updeep automatically merges the nested name
object, preserving the last
name.
Updating Arrays with Precision
Updeep’s magic extends to arrays as well. Let’s see how we can update specific elements:
const scores = [10, 20, 30, 40];
const updatedScores = u({ 1: 25, 3: 45 }, scores);
console.log(updatedScores); // Output: [10, 25, 30, 45]
Advanced Sorcery
Now that we’ve mastered the basics, let’s explore some more advanced techniques.
The Map Spell
The u.map
function allows you to apply updates to every element of an array or object:
const students = [
{ name: 'Luna', house: 'Ravenclaw' },
{ name: 'Neville', house: 'Gryffindor' }
];
const graduatedStudents = u.map({ status: 'graduated' }, students);
console.log(graduatedStudents);
// Output: [
// { name: 'Luna', house: 'Ravenclaw', status: 'graduated' },
// { name: 'Neville', house: 'Gryffindor', status: 'graduated' }
// ]
Conditional Updates with If and IfElse
Updeep provides u.if
and u.ifElse
for conditional updates:
const character = { name: 'Draco', house: 'Slytherin', isEvil: true };
const reformedCharacter = u({
isEvil: u.ifElse(
(isEvil) => isEvil === true,
false,
(currentValue) => currentValue
)
}, character);
console.log(reformedCharacter); // Output: { name: 'Draco', house: 'Slytherin', isEvil: false }
Omitting Properties
Sometimes, you need to remove properties. Updeep’s u.omit
comes to the rescue:
const secretInfo = {
name: 'Severus Snape',
role: 'Professor',
secretAllegiance: 'Order of the Phoenix'
};
const publicInfo = u({
secretAllegiance: u.omitted
}, secretInfo);
console.log(publicInfo); // Output: { name: 'Severus Snape', role: 'Professor' }
Mastering the Art of Immutable Updates
As we’ve seen, updeep provides a powerful set of tools for managing immutable state in React applications. Its declarative approach and ability to handle deeply nested structures make it an invaluable asset in any developer’s toolkit.
By leveraging updeep, you can:
- Simplify complex state updates
- Improve performance through efficient immutable operations
- Write more readable and maintainable code
- Reduce the risk of accidental state mutations
As you continue your journey with updeep, remember that its true power lies in its composability and flexibility. Experiment with combining different updeep functions, and you’ll find yourself casting ever more sophisticated spells of state management.
For those interested in exploring other state management solutions, you might want to check out our article on Mastering Jotai React State, which offers an alternative approach to managing React state.
In the grand tapestry of React development, updeep stands out as a thread of pure magic, weaving immutability and simplicity into the very fabric of your applications. So go forth, wield your new-found powers responsibly, and may your state always remain pristine and immutable!
If you’re looking to further enhance your React development skills, don’t miss our guide on Mastering React Datepicker, which can complement your state management strategies with powerful date handling capabilities.