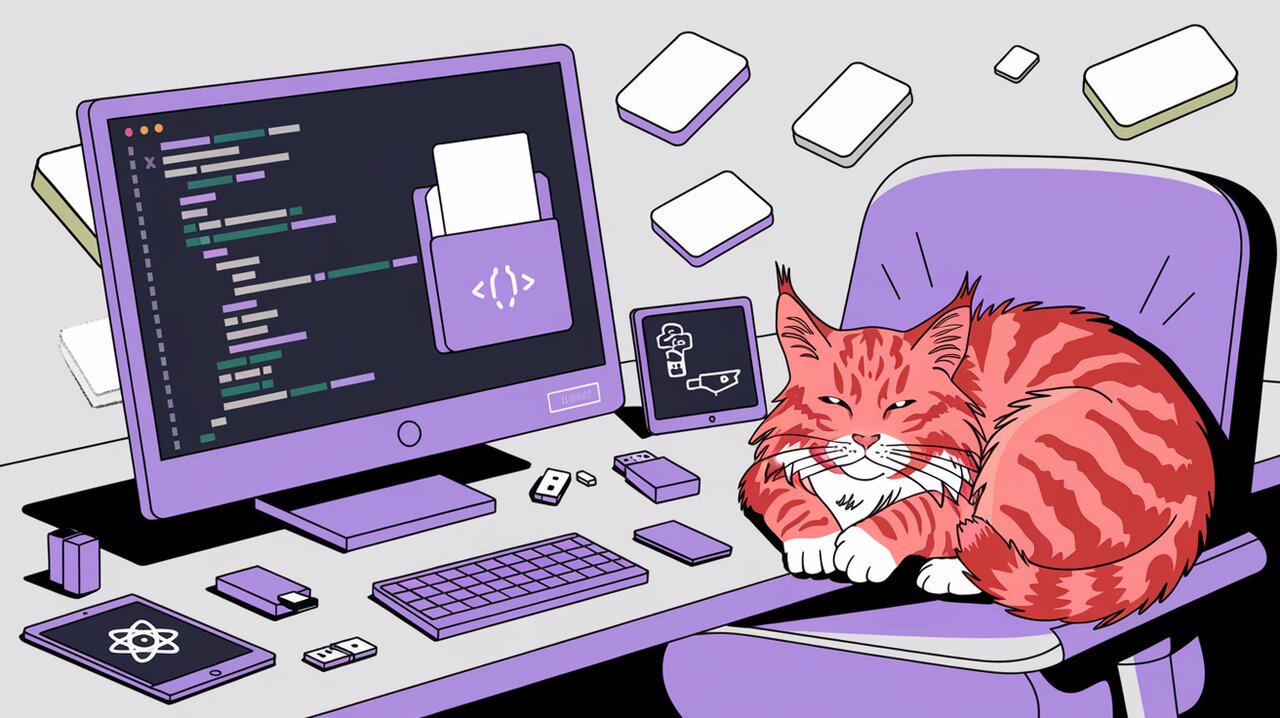
Uploady: Streamlining File Uploads in React with Ease
@
rpldy/uploady` is a modern, lightweight library designed to simplify file uploads in React applications. With its modular architecture and extensive feature set, Uploady empowers developers to create efficient and customizable file upload experiences with minimal code.
Features
- Lightweight Core: Uploady’s core is small and efficient, with minimal dependencies.
- Modular Design: Choose only the components and features you need.
- Customizable: Extensive configuration options for fine-tuning the upload process.
- Hooks-based API: Leverage React hooks for easy integration and state management.
- UI Components: Ready-to-use components like upload buttons and drop zones.
- Advanced Capabilities: Support for chunked uploads, retry mechanisms, and more.
Installation
To get started with Uploady, you’ll need to install the core package and any additional modules you require. Here’s how to install the main Uploady provider:
# Using npm
npm install @rpldy/uploady
# Using Yarn
yarn add @rpldy/uploady
For projects that only need the upload mechanism without UI components, you can install the Uploader package:
# Using npm
npm install @rpldy/uploader
# Using Yarn
yarn add @rpldy/uploader
Basic Usage
Simple Upload Button
Let’s start with a basic example of how to implement a file upload button using Uploady:
import React from "react";
import Uploady from "@rpldy/uploady";
import UploadButton from "@rpldy/upload-button";
const App: React.FC = () => (
<Uploady
destination={{ url: "https://my-server.com/upload" }}
>
<UploadButton />
</Uploady>
);
export default App;
In this example, we wrap our application (or a part of it) with the Uploady
provider and use the UploadButton
component to create a clickable upload button. The destination
prop specifies where the files will be uploaded.
Customizing the Upload Button
You can easily customize the appearance and behavior of the upload button:
import React from "react";
import Uploady from "@rpldy/uploady";
import UploadButton from "@rpldy/upload-button";
const App: React.FC = () => (
<Uploady
destination={{ url: "https://my-server.com/upload" }}
multiple={true}
accept="image/*"
>
<UploadButton text="Upload Images">
<span>Select Images to Upload</span>
</UploadButton>
</Uploady>
);
export default App;
This example adds support for multiple file selection, restricts uploads to image files, and customizes the button text.
Advanced Usage
Drag and Drop Uploads
Uploady makes it easy to implement drag and drop file uploads:
import React from "react";
import Uploady from "@rpldy/uploady";
import UploadDropZone from "@rpldy/upload-drop-zone";
const App: React.FC = () => (
<Uploady
destination={{ url: "https://my-server.com/upload" }}
>
<UploadDropZone
onDragOverClassName="drag-over"
extraProps={{ style: { width: 600, height: 400 } }}
>
<span>Drag and Drop Files Here</span>
</UploadDropZone>
</Uploady>
);
export default App;
This creates a drop zone where users can drag and drop files to upload. The onDragOverClassName
prop allows you to style the drop zone when files are dragged over it.
Upload Preview
Uploady provides a convenient way to show previews of files being uploaded:
import React from "react";
import Uploady from "@rpldy/uploady";
import UploadPreview from "@rpldy/upload-preview";
const App: React.FC = () => (
<Uploady
destination={{ url: "https://my-server.com/upload" }}
>
<UploadPreview
rememberPreviousBatches
fallbackUrl="https://icon-library.com/images/file-icon-png/file-icon-png-25.jpg"
/>
<UploadButton />
</Uploady>
);
export default App;
This example adds a preview component that shows thumbnails of uploaded files. The rememberPreviousBatches
prop ensures that previews from previous upload batches are retained, and fallbackUrl
provides a default image for non-previewable files.
Chunked Uploads
For large files, Uploady supports chunked uploads:
import React from "react";
import ChunkedUploady from "@rpldy/chunked-uploady";
import UploadButton from "@rpldy/upload-button";
const App: React.FC = () => (
<ChunkedUploady
destination={{ url: "https://my-server.com/upload" }}
chunkSize={5242880} // 5MB chunks
>
<UploadButton />
</ChunkedUploady>
);
export default App;
This setup uses ChunkedUploady
to enable chunked uploads, with each chunk set to 5MB. This is particularly useful for uploading large files and can help improve upload reliability.
Using Hooks for Custom Behavior
Uploady provides various hooks that allow you to customize the upload process:
import React from "react";
import Uploady, { useItemStartListener } from "@rpldy/uploady";
import UploadButton from "@rpldy/upload-button";
const UploadLogger: React.FC = () => {
useItemStartListener((item) => {
console.log(`Starting to upload ${item.file.name}`);
});
return null;
};
const App: React.FC = () => (
<Uploady
destination={{ url: "https://my-server.com/upload" }}
>
<UploadLogger />
<UploadButton />
</Uploady>
);
export default App;
In this example, we use the useItemStartListener
hook to log a message when each file starts uploading. This demonstrates how you can use Uploady’s hooks to add custom behaviors to your upload process.
Conclusion
@rpldy/uploady
offers a powerful and flexible solution for handling file uploads in React applications. Its modular design allows developers to choose only the features they need, keeping the bundle size small. With support for advanced features like chunked uploads, drag and drop, and customizable UI components, Uploady can handle a wide range of upload scenarios.
By leveraging Uploady’s hooks and components, developers can create sophisticated upload interfaces with minimal code. Whether you’re building a simple file upload button or a complex file management system, Uploady provides the tools you need to streamline the process and enhance the user experience.
As you dive deeper into Uploady, you’ll discover even more features and customization options that can help you tailor the upload experience to your specific needs. Happy uploading!