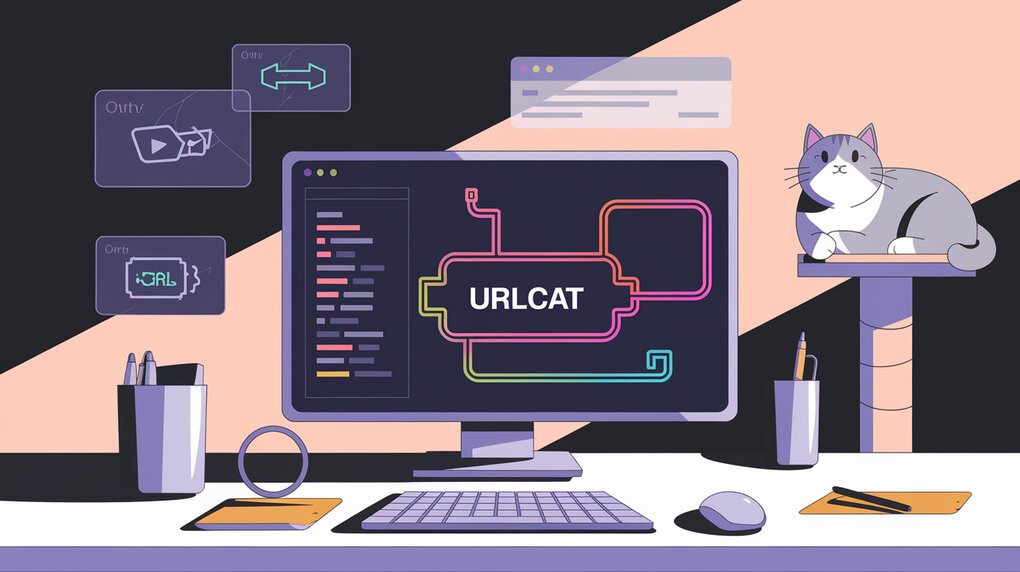
Urlcat is a tiny JavaScript library that revolutionizes the way developers handle URL construction in React applications. By providing a simple and intuitive API, urlcat helps prevent common mistakes and makes building URLs a breeze. Whether you’re working with REST APIs, constructing dynamic routes, or handling complex query parameters, urlcat has got you covered.
Features
Urlcat comes packed with features that make it stand out from other URL-building solutions:
- Lightweight: At just 10.5KB minified and gzipped, urlcat won’t bloat your application.
- TypeScript support: First-class TypeScript types are provided out of the box.
- Flexible API: Handles both path and query parameters with ease.
- Error prevention: Automatically takes care of URL encoding and edge cases.
- Minimal dependencies: Only one dependency, keeping your project lean.
Installation
Getting started with urlcat is straightforward. You can install it using npm or yarn:
# Using npm
npm install urlcat
# Using yarn
yarn add urlcat
Basic Usage
Let’s dive into some basic examples of how to use urlcat in your React applications.
Simple URL Construction
To create a simple URL with query parameters:
import urlcat from 'urlcat';
const apiUrl = urlcat('https://api.example.com', '/users', { limit: 10, offset: 20 });
console.log(apiUrl);
// Output: 'https://api.example.com/users?limit=10&offset=20'
Path Parameters
Urlcat shines when dealing with path parameters:
const userPostUrl = urlcat('https://api.example.com', '/users/:id/posts', { id: 123, sort: 'latest' });
console.log(userPostUrl);
// Output: 'https://api.example.com/users/123/posts?sort=latest'
Handling Special Characters
Urlcat automatically takes care of URL encoding:
const searchUrl = urlcat('https://example.com', '/search', { q: 'React & TypeScript' });
console.log(searchUrl);
// Output: 'https://example.com/search?q=React%20%26%20TypeScript'
Advanced Usage
Now let’s explore some more advanced features of urlcat.
Combining Path and Query Parameters
Urlcat seamlessly handles a mix of path and query parameters:
const complexUrl = urlcat('https://api.example.com', '/users/:userId/posts/:postId/comments', {
userId: 123,
postId: 456,
sort: 'newest',
limit: 50
});
console.log(complexUrl);
// Output: 'https://api.example.com/users/123/posts/456/comments?sort=newest&limit=50'
Working with Arrays
When dealing with array parameters, urlcat has you covered:
const filterUrl = urlcat('https://api.example.com', '/products', {
category: ['electronics', 'accessories'],
brand: 'apple'
});
console.log(filterUrl);
// Output: 'https://api.example.com/products?category=electronics&category=accessories&brand=apple'
Custom Separators
For more control over URL construction, you can use the join
function:
import { join } from 'urlcat';
const customUrl = join('api', '/', 'v2', '/', 'users');
console.log(customUrl);
// Output: 'api/v2/users'
TypeScript Enhancements
Urlcat provides excellent TypeScript support, allowing you to define strict types for your URL parameters:
interface UserParams {
id: number;
role: 'admin' | 'user';
}
const userUrl = urlcat<UserParams>('https://api.example.com', '/users/:id', { id: 123, role: 'admin' });
console.log(userUrl);
// Output: 'https://api.example.com/users/123?role=admin'
Conclusion
Urlcat proves to be an invaluable tool for React developers working with URLs. Its simple yet powerful API, combined with automatic error prevention and TypeScript support, makes it a top choice for URL manipulation tasks. By using urlcat, you can significantly reduce the complexity of URL handling in your React applications, leading to cleaner, more maintainable code.
Whether you’re building a small project or a large-scale application, urlcat’s flexibility and ease of use make it a purr-fect addition to your development toolkit. Give it a try in your next React project and experience the difference it can make in simplifying your URL-related code.