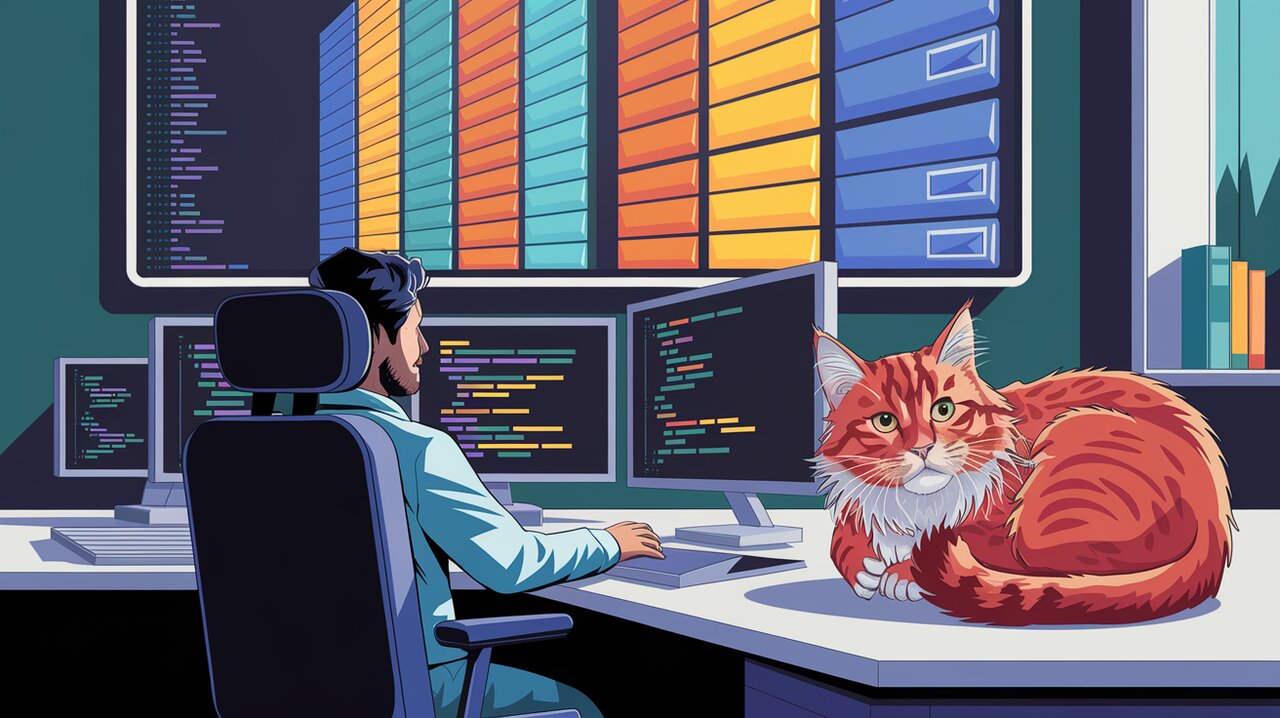
Supercharge Your React Lists: Unleash the Power of react-tiny-virtual-list
Introducing react-tiny-virtual-list
In the realm of React development, efficiently rendering large lists of data is a common challenge. Enter react-tiny-virtual-list
, a compact yet powerful library that revolutionizes the way we handle extensive datasets in our applications. With a mere 3kb gzipped footprint and zero dependencies, this library packs a punch when it comes to list virtualization.
Key Features
react-tiny-virtual-list
boasts an impressive array of features that make it a go-to solution for React developers:
- Lightweight: At just 3kb gzipped, it won’t bloat your application.
- Zero Dependencies: Standalone functionality without relying on external libraries.
- Millions of Items: Render vast amounts of data without performance hiccups.
- Flexible Heights: Support for both fixed and variable item heights.
- Scroll Control: Easily scroll to specific indices or set initial scroll offsets.
- Bi-directional: Works with both vertical and horizontal lists.
Getting Started
Installation
To begin using react-tiny-virtual-list
, you’ll need to install it in your project. You can do this using npm or yarn:
npm install react-tiny-virtual-list --save
or
yarn add react-tiny-virtual-list
Basic Usage
Let’s dive into a simple example to demonstrate how easy it is to use react-tiny-virtual-list
:
import React from 'react';
import VirtualList from 'react-tiny-virtual-list';
const MyList = () => {
const data = ['A', 'B', 'C', 'D', 'E', 'F', /* ... */];
return (
<VirtualList
width="100%"
height={600}
itemCount={data.length}
itemSize={50}
renderItem={({index, style}) => (
<div key={index} style={style}>
Letter: {data[index]}, Row: #{index}
</div>
)}
/>
);
};
In this example, we create a virtual list that renders a large dataset of letters. The VirtualList
component takes care of efficiently rendering only the visible items, greatly improving performance for large lists.
Advanced Usage
Variable Heights
react-tiny-virtual-list
supports items with variable heights, which is crucial for rendering content of different sizes:
const MyVariableHeightList = () => {
const getItemSize = (index: number) => {
// Return different heights based on the index
return index % 2 === 0 ? 50 : 75;
};
return (
<VirtualList
width="100%"
height={600}
itemCount={1000}
itemSize={getItemSize}
renderItem={({index, style}) => (
<div key={index} style={style}>
Item #{index}
</div>
)}
/>
);
};
This example demonstrates how to use a function to determine the height of each item dynamically.
Horizontal Lists
Creating horizontal lists is just as simple:
const MyHorizontalList = () => {
return (
<VirtualList
width={600}
height={50}
itemCount={1000}
itemSize={100}
horizontal={true}
renderItem={({index, style}) => (
<div key={index} style={style}>
Column #{index}
</div>
)}
/>
);
};
By setting the horizontal
prop to true
, we create a horizontally scrolling list.
Scroll to Index
react-tiny-virtual-list
allows you to programmatically scroll to a specific index:
const MyScrollableList = () => {
const listRef = React.useRef(null);
const handleScrollToIndex = () => {
listRef.current.scrollToIndex(50);
};
return (
<>
<button onClick={handleScrollToIndex}>Scroll to Index 50</button>
<VirtualList
ref={listRef}
width="100%"
height={600}
itemCount={1000}
itemSize={50}
renderItem={({index, style}) => (
<div key={index} style={style}>
Item #{index}
</div>
)}
/>
</>
);
};
This example shows how to use the scrollToIndex
method to navigate to a specific item in the list.
Performance Considerations
While react-tiny-virtual-list
is highly optimized, there are a few things to keep in mind for optimal performance:
- Memoize Render Functions: Use
React.memo
oruseMemo
to prevent unnecessary re-renders of list items. - Avoid Complex Calculations: Keep the
renderItem
function as simple as possible to ensure smooth scrolling. - Optimize Images: If rendering images, consider lazy loading techniques to further improve performance.
Conclusion
react-tiny-virtual-list
is a powerful tool in the React developer’s arsenal for handling large datasets efficiently. Its small size, zero dependencies, and robust feature set make it an excellent choice for projects of all sizes. By leveraging this library, you can create smooth, performant list experiences that can handle millions of items without breaking a sweat.
Whether you’re building a complex data grid or a simple scrollable list, react-tiny-virtual-list
provides the flexibility and performance you need to create outstanding user interfaces. Give it a try in your next project and experience the power of efficient list virtualization in React!