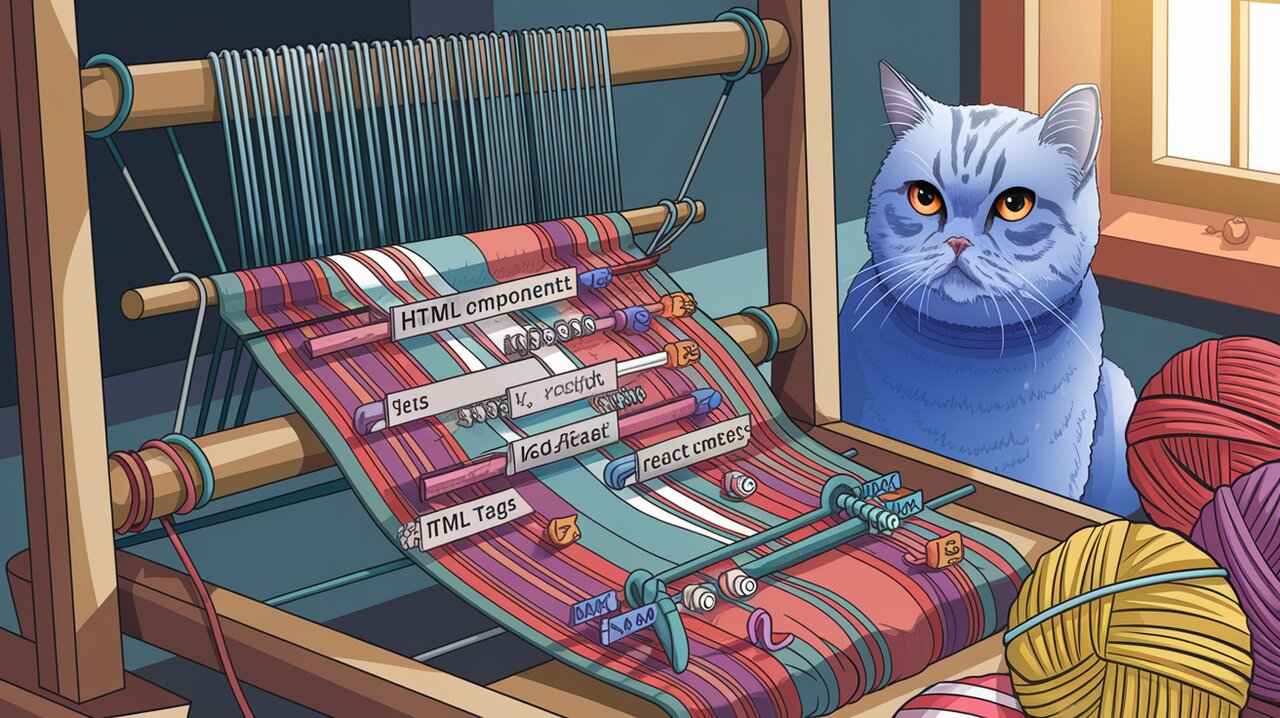
Weaving Magic: Interweave Your React Apps with Safe HTML Rendering
In the ever-evolving landscape of React development, handling dynamic HTML content safely and efficiently can be a daunting task. Enter Interweave, a powerful library that revolutionizes the way we render HTML, filter attributes, and manipulate text within React applications. This versatile tool not only addresses common security concerns but also provides a rich set of features to enhance your content rendering capabilities.
Unraveling Interweave’s Capabilities
Interweave brings a plethora of features to the table, making it an indispensable tool for React developers:
-
Safe HTML Rendering: Say goodbye to the risks associated with
dangerouslySetInnerHTML
. Interweave allows you to render HTML content securely. -
XSS Protection: Built-in safeguards against cross-site scripting attacks keep your application secure.
-
HTML Attribute Filtering: Clean and sanitize HTML attributes with ease using customizable filters.
-
Component Interpolation: Seamlessly integrate React components within your HTML content using matchers.
-
Autolinking: Automatically convert URLs, IP addresses, email addresses, and hashtags into clickable links.
-
Emoji Rendering: Bring your content to life with support for emoji and emoticon characters.
-
HTML Tag Stripping: When needed, safely remove HTML tags from your content.
Weaving Interweave Into Your Project
Before we dive into the magic of Interweave, let’s get it set up in your React project.
Installation
You can easily add Interweave to your project using npm or yarn:
npm install interweave react
# Or if you prefer yarn
yarn add interweave react
Make sure you have React 16.8+ or 17+ installed, as Interweave requires it as a peer dependency.
Basic Usage: Your First Interweave
Let’s start with a simple example to demonstrate how Interweave renders HTML content safely.
import React from 'react';
import { Interweave } from 'interweave';
const MyComponent = () => {
const htmlContent = "<p>Welcome to <strong>Interweave</strong>!</p>";
return <Interweave content={htmlContent} />;
};
In this example, Interweave safely renders the HTML content without using dangerouslySetInnerHTML
. The <p>
and <strong>
tags are preserved, and the content is displayed as intended.
Autolinking URLs and Hashtags
One of Interweave’s powerful features is its ability to automatically convert URLs and hashtags into clickable links. Here’s how you can use it:
import React from 'react';
import { Interweave, UrlMatcher, HashtagMatcher } from 'interweave';
const SocialContent = () => {
const content = "Check out https://example.com and follow #interweave!";
return (
<Interweave
content={content}
matchers={[new UrlMatcher('url'), new HashtagMatcher('hashtag')]}
/>
);
};
This code will render the URL as a clickable link and the hashtag as a link that could be used for searching or filtering.
Advanced Techniques: Unleashing Interweave’s Full Potential
Now that we’ve covered the basics, let’s explore some of Interweave’s more advanced features.
Custom Matchers: Tailoring Content to Your Needs
Interweave allows you to create custom matchers to transform specific patterns in your content. Here’s an example of a custom matcher that highlights user mentions:
import React from 'react';
import { Interweave, Matcher } from 'interweave';
class UserMentionMatcher extends Matcher {
asTag(): string {
return 'a';
}
match(string: string) {
return this.doMatch(string, /@(\w+)/, (matches) => ({
match: matches[0],
username: matches[1],
}));
}
replaceWith(match: string, props: { username: string }) {
return (
<a href={`/user/${props.username}`} className="user-mention">
{match}
</a>
);
}
}
const UserContent = () => {
const content = "Hello @johndoe, welcome to our community!";
return (
<Interweave
content={content}
matchers={[new UserMentionMatcher('mention')]}
/>
);
};
This custom matcher will transform @username
mentions into clickable links to user profiles.
Filtering Attributes: Ensuring Content Safety
Interweave provides robust attribute filtering to prevent XSS attacks and ensure content safety. Here’s how you can use attribute filters:
import React from 'react';
import { Interweave, FilterFactory } from 'interweave';
const customFilter = FilterFactory.createAttributeFilter(['id', 'class'], {
'a': ['href', 'target'],
'img': ['src', 'alt'],
});
const SafeContent = () => {
const content = `
<a href="https://example.com" onclick="alert('XSS')">Safe Link</a>
<img src="image.jpg" onerror="alert('XSS')" alt="Safe Image" />
`;
return <Interweave content={content} filters={[customFilter]} />;
};
This example demonstrates how to create a custom attribute filter that only allows specific attributes on certain tags, effectively removing potentially harmful attributes like onclick
and onerror
.
Emoji Rendering: Adding Expressiveness to Your Content
Interweave can also handle emoji rendering, bringing life to your text content:
import React from 'react';
import { Interweave, EmojiMatcher } from 'interweave';
import data from 'emojibase-data/en/data.json';
const EmojiContent = () => {
const content = "I love using Interweave! :heart: It's so cool! :sunglasses:";
return (
<Interweave
content={content}
matchers={[new EmojiMatcher('emoji', { convertEmoticon: true, convertShortcode: true })]}
emojiSource={(hex) => `https://cdn.jsdelivr.net/npm/emoji-datasource-apple@6.0.1/img/apple/64/${hex}.png`}
emojiPath="https://cdn.jsdelivr.net/npm/emoji-datasource-apple@6.0.1/img/apple/64/"
emojiSize={16}
/>
);
};
This example shows how to use the EmojiMatcher
to convert both emoji shortcodes and emoticons into actual emoji images.
Wrapping Up: The Power of Interweave
Interweave is more than just an HTML renderer for React; it’s a comprehensive solution for handling complex content scenarios safely and efficiently. From its core feature of secure HTML rendering to advanced capabilities like custom matchers and attribute filtering, Interweave provides the tools you need to create rich, interactive, and secure content experiences in your React applications.
By leveraging Interweave’s features, you can focus on creating engaging content without worrying about the underlying complexities of HTML parsing, security, and content transformation. Whether you’re building a social media platform, a content management system, or any application that deals with user-generated content, Interweave offers the flexibility and robustness to meet your needs.
As you continue to explore Interweave, you’ll discover even more ways to enhance your React applications, making content rendering a seamless and secure part of your development process. Happy weaving!