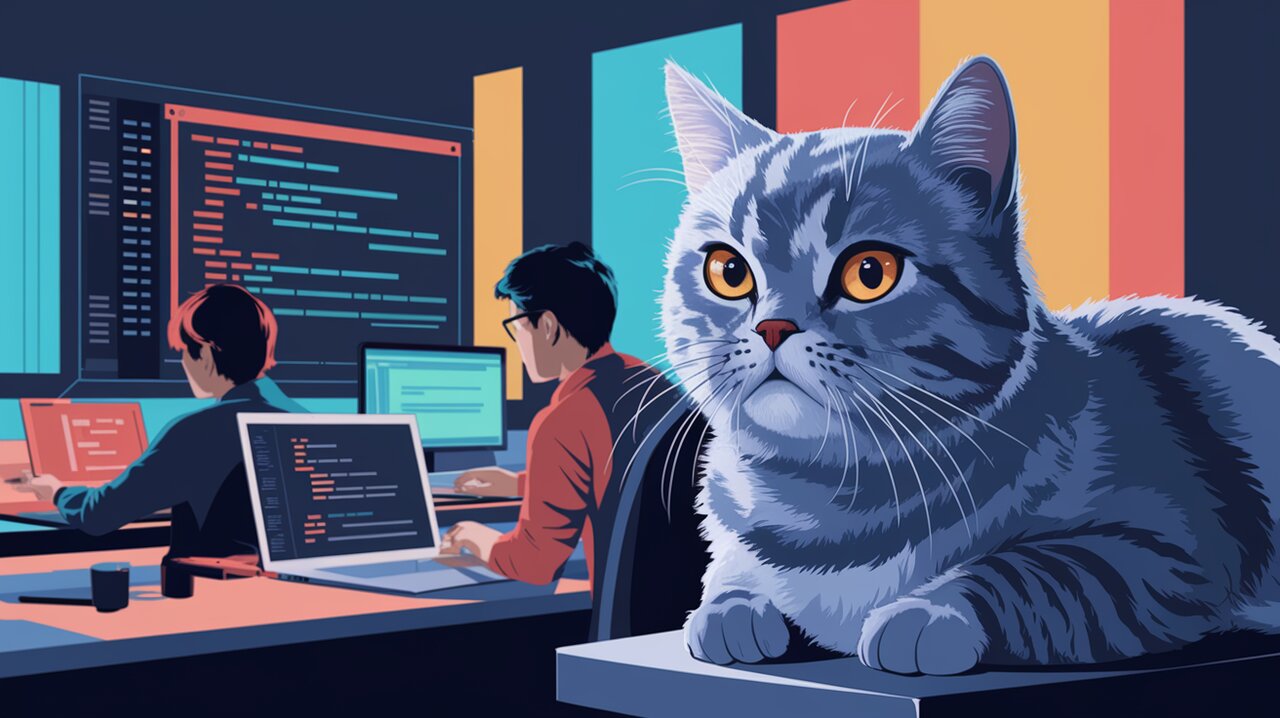
Whip Up Streaming Magic with react-native-whip-whep
React Native has become a cornerstone for building cross-platform mobile applications, and with the introduction of react-native-whip-whep, developers can now integrate advanced media streaming capabilities using WebRTC protocols. This library is designed to implement WHIP (WebRTC-HTTP Ingestion Protocol) and WHEP (WebRTC-HTTP Egress Protocol), facilitating seamless media streaming in React Native apps. Whether you’re building a live broadcasting app or a video conferencing tool, react-native-whip-whep
offers robust solutions for your streaming needs.
Key Features
- Cross-Platform Compatibility: Works seamlessly on both Android and iOS platforms.
- Native Views: Provides
WhepClientView
andWhipClientView
for displaying streams and camera previews. - Simple Integration: Easily establish WebRTC PeerConnections to send or receive media streams.
Getting Started
To begin using react-native-whip-whep
, you can install it via npm or yarn:
npm install --save react-native-whip-whep
# OR
yarn add react-native-whip-whep
Configuration
With Expo
If you’re using Expo, update your app.json
to include necessary permissions:
Android
{
"expo": {
"android": {
"permissions": [
"android.permission.CAMERA",
"android.permission.RECORD_AUDIO"
]
}
}
}
iOS
{
"expo": {
"ios": {
"infoPlist": {
"NSCameraUsageDescription": "This application requires camera access.",
"NSMicrophoneUsageDescription": "This application requires microphone access."
}
}
}
}
Without Expo
For bare React Native projects, ensure you have expo-modules
installed:
npx install-expo-modules
Update your AndroidManifest.xml and info.plist files similarly to configure permissions.
Basic Usage
Setting Up a WHEP Client
To receive media streams, create a WHEP client:
import { createWhepClient, connectWhepClient, disconnectWhepClient } from 'react-native-whip-whep';
const initializeWhep = async () => {
await createWhepClient('https://your.whep.server.url', { authToken: 'your_auth_token' });
};
const startReceivingStream = async () => {
await connectWhepClient();
};
// Call this function when you want to stop receiving the stream.
const stopReceivingStream = () => {
disconnectWhepClient();
};
Displaying Streams
Use WhepClientView
to display the received stream in your component:
import { WhepClientView } from 'react-native-whip-whep';
const StreamViewer = () => (
<WhepClientView style={{ width: '100%', height: '100%' }} />
);
Advanced Usage
Creating a WHIP Client for Streaming
To broadcast media streams, set up a WHIP client:
import { createWhipClient, connectWhipClient, disconnectWhipClient, cameras } from 'react-native-whip-whep';
const availableDevices = cameras;
const initializeWhip = async () => {
await createWhipClient('https://your.whip.server.url', { authToken: 'your_auth_token' }, availableDevices[0].id);
};
const startBroadcasting = async () => {
await connectWhipClient();
};
// Call this function when you want to stop broadcasting.
const stopBroadcasting = () => {
disconnectWhipClient();
};
Customizing Media Parameters
You can customize video parameters or choose different STUN servers during client creation:
createWhipClient('https://your.whip.server.url', {
authToken: 'your_auth_token',
videoParameters: { resolution: '720p' },
}, availableDevices[0].id);
Wrapping Up
The react-native-whip-whep library is an excellent tool for developers looking to enhance their React Native applications with powerful media streaming capabilities. By leveraging WHIP and WHEP protocols, you can build sophisticated applications that handle both media ingestion and egress efficiently. Whether you’re aiming to develop live streaming platforms or interactive communication apps, this library provides the necessary building blocks to get started quickly and effectively.