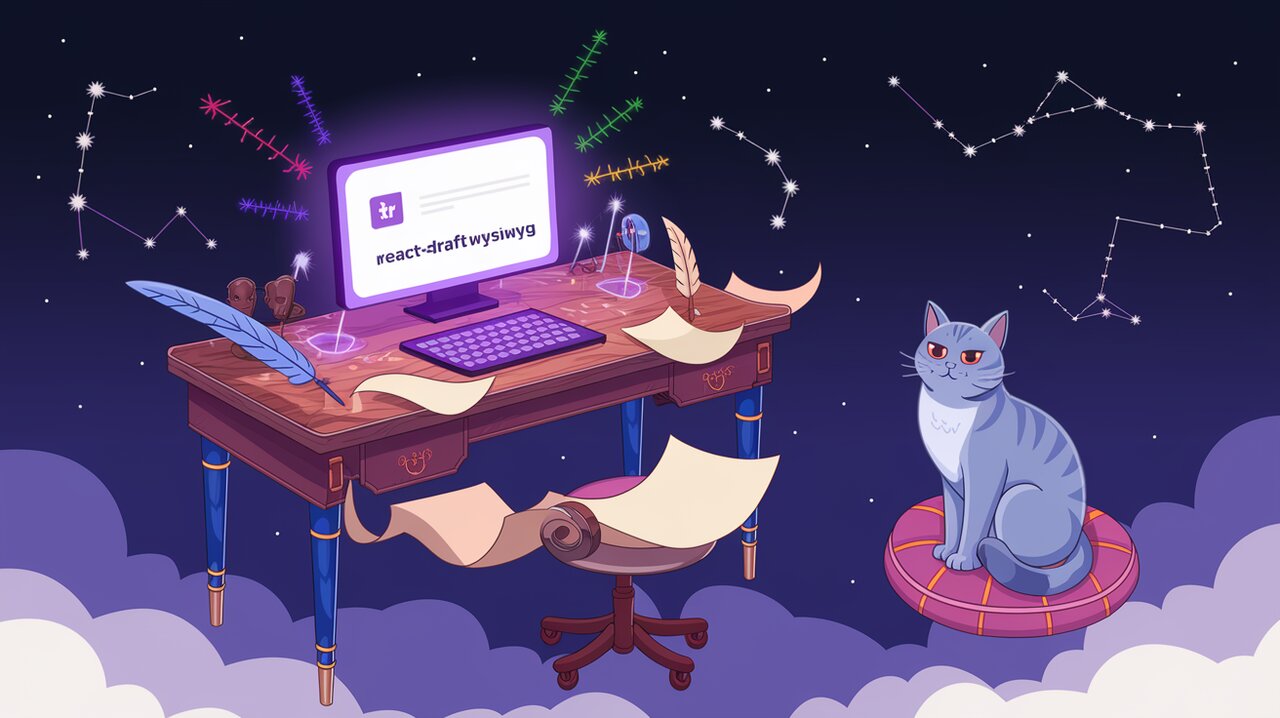
WYSIWYG Wizardry: Conjuring Rich Text Editors with React-Draft-Wysiwyg
React-Draft-Wysiwyg is a powerful and flexible WYSIWYG (What You See Is What You Get) editor built for React applications. It harnesses the capabilities of Draft.js, a rich text editor framework developed by Facebook, to provide a seamless and intuitive editing experience. Whether you’re crafting a blog platform, a content management system, or any application that requires rich text input, React-Draft-Wysiwyg equips you with the tools to create stunning, formatted content with ease.
Unveiling the Magic: Key Features
React-Draft-Wysiwyg comes packed with an array of features that make it a standout choice for developers:
- Customizable Toolbar: Tailor the editor’s toolbar to your needs by adding, removing, or reordering controls.
- Rich Text Formatting: Support for inline styles like bold, italic, underline, and more.
- Block-Level Formatting: Easily create headings, blockquotes, and code blocks.
- Lists and Indentation: Organize content with ordered and unordered lists, complete with indentation support.
- Text Alignment: Align text to left, right, center, or justify with ease.
- Color Magic: Apply colors to text or background for vibrant content.
- Link Enchantment: Add and edit links effortlessly.
- Emoji Spells: Choose from over 150 emojis to express emotions.
- Mention Charms: Implement mentions for user tagging.
- Hashtag Hexes: Add support for hashtags in your content.
- Image Sorcery: Upload, resize, and align images within your content.
- Embedded Incantations: Flexibility to embed external content with customizable dimensions.
- Undo/Redo Wizardry: Never fear mistakes with undo and redo functionality.
- Internationalization Illusions: Support for multiple languages to cater to a global audience.
Summoning the Editor: Installation
To begin your journey with React-Draft-Wysiwyg, you’ll need to summon it into your project. Open your magical terminal and cast these spells:
npm install --save react-draft-wysiwyg draft-js
# or if you prefer yarn
yarn add react-draft-wysiwyg draft-js
Casting Your First Spell: Basic Usage
Now that you’ve summoned the library, it’s time to cast your first spell. Here’s a basic incantation to conjure a simple editor:
import React, { useState } from 'react';
import { EditorState } from 'draft-js';
import { Editor } from 'react-draft-wysiwyg';
import 'react-draft-wysiwyg/dist/react-draft-wysiwyg.css';
const MyWysiwygEditor: React.FC = () => {
const [editorState, setEditorState] = useState(EditorState.createEmpty());
const onEditorStateChange = (newEditorState: EditorState) => {
setEditorState(newEditorState);
};
return (
<div>
<Editor
editorState={editorState}
onEditorStateChange={onEditorStateChange}
wrapperClassName="demo-wrapper"
editorClassName="demo-editor"
/>
</div>
);
};
export default MyWysiwygEditor;
This spell creates a basic editor with default settings. The editorState
holds the current content and formatting of the editor, while onEditorStateChange
updates this state as the user types or formats text.
Advanced Incantations: Customizing Your Editor
Toolbar Transmutation
To customize the toolbar, you can pass a toolbar
prop to the Editor
component. This allows you to specify which controls appear and in what order:
const MyCustomEditor: React.FC = () => {
// ... previous state setup
return (
<Editor
editorState={editorState}
onEditorStateChange={onEditorStateChange}
toolbar={{
options: ['inline', 'blockType', 'list', 'textAlign', 'link', 'emoji'],
inline: { options: ['bold', 'italic', 'underline'] },
list: { options: ['unordered', 'ordered'] },
}}
/>
);
};
This configuration creates a streamlined toolbar with only inline formatting, block types, lists, text alignment, links, and emojis.
Image Enchantment
To allow users to upload images, you can provide an uploadCallback
function:
const uploadImageCallBack = (file: File): Promise<{ data: { link: string } }> => {
return new Promise((resolve, reject) => {
const reader = new FileReader();
reader.onload = () => resolve({ data: { link: reader.result as string } });
reader.onerror = error => reject(error);
reader.readAsDataURL(file);
});
};
const MyImageEnabledEditor: React.FC = () => {
// ... previous state setup
return (
<Editor
editorState={editorState}
onEditorStateChange={onEditorStateChange}
toolbar={{
image: {
uploadCallback: uploadImageCallBack,
alt: { present: true, mandatory: false },
},
}}
/>
);
};
This spell allows users to upload images directly into the editor, with options for adding alt text.
Mention Magic
To add mention functionality, you can configure the mention option in the toolbar:
const mentions = [
{ text: 'Merlin', value: 'merlin', url: 'https://en.wikipedia.org/wiki/Merlin' },
{ text: 'Gandalf', value: 'gandalf', url: 'https://en.wikipedia.org/wiki/Gandalf' },
// ... more wizards
];
const MyMentionEnabledEditor: React.FC = () => {
// ... previous state setup
return (
<Editor
editorState={editorState}
onEditorStateChange={onEditorStateChange}
toolbar={{
options: ['inline', 'blockType', 'list', 'link', 'image', 'history'],
mention: {
separator: ' ',
trigger: '@',
suggestions: mentions,
},
}}
/>
);
};
This configuration allows users to mention wizards by typing ’@’ followed by their name.
Conclusion: Mastering the Art of WYSIWYG
React-Draft-Wysiwyg provides a powerful set of tools for creating rich, interactive content editors in your React applications. From basic text formatting to advanced features like image uploading and mentions, this library offers the flexibility to craft the perfect editing experience for your users.
As you continue your journey into the realm of WYSIWYG editing, you might want to explore related enchantments. Consider delving into the world of markdown editing with “Simplify Markdown Editing: React SimpleMDE” or explore alternative rich text editing solutions with “Unleashing Slate’s Power: React Rich Text Editing”. These articles can be found in the arcane tomes of our site, offering complementary knowledge to expand your text editing wizardry.
Remember, the true power of React-Draft-Wysiwyg lies in its customizability. Experiment with different configurations, add your own magical touches, and create an editing experience that truly captivates your users. Happy spell-casting!