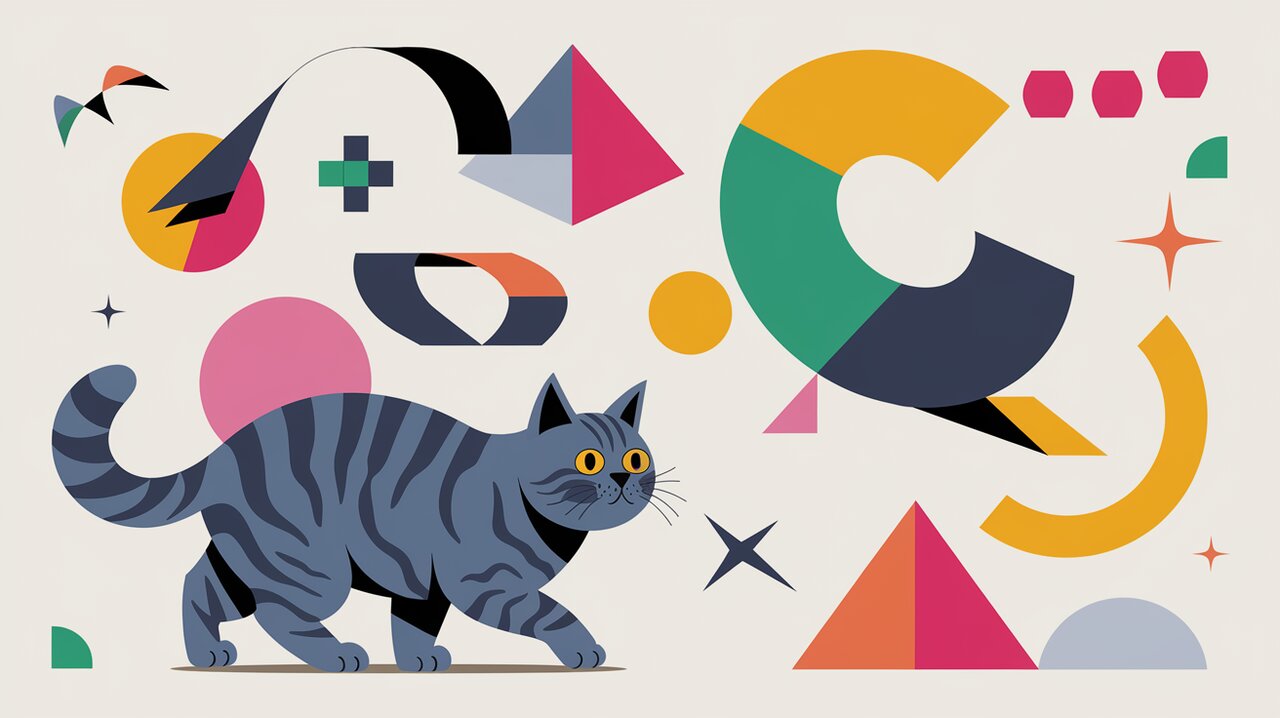
Skia Symphony: Unleashing 2D Graphics in React Native
React Native Skia brings the power of the Skia Graphics Library to React Native, enabling developers to create high-performance 2D graphics and animations. This library serves as the graphics engine for popular products like Google Chrome, Android, Flutter, and Mozilla Firefox. With React Native Skia, you can elevate your mobile app’s visual appeal and performance to new heights.
Features
React Native Skia comes packed with an array of powerful features:
- High-performance rendering: Leverage the speed and efficiency of the Skia engine for smooth graphics and animations.
- Cross-platform compatibility: Create consistent visuals across iOS and Android platforms.
- Rich set of drawing primitives: Access a wide range of shapes, paths, and effects for complex designs.
- Hardware acceleration: Utilize GPU capabilities for enhanced performance.
- Declarative API: Enjoy the familiar React programming model for creating graphics.
Installation
To get started with React Native Skia, you’ll need to install it in your React Native project. Here’s how you can do it using npm or yarn:
npm install @shopify/react-native-skia
or
yarn add @shopify/react-native-skia
After installation, you’ll need to link the native modules. For React Native 0.60 and above, this should happen automatically. For earlier versions, you might need to run:
react-native link @shopify/react-native-skia
Basic Usage
Let’s dive into some basic examples to get you started with React Native Skia.
Drawing a Simple Shape
Here’s how you can draw a simple circle using React Native Skia:
import {Canvas, Circle, Fill} from "@shopify/react-native-skia";
export const SimpleCircle = () => {
const size = 256;
const r = size * 0.33;
return (
<Canvas style={{ flex: 1 }}>
<Fill color="white" />
<Circle cx={r} cy={r} r={r} color="lightblue" />
</Canvas>
);
};
In this example, we create a canvas and draw a light blue circle on it. The Canvas
component serves as the drawing area, while the Circle
component defines our shape.
Creating Animations
React Native Skia shines when it comes to animations. Here’s a simple example of an animated circle:
import {Canvas, Circle, useValue, useComputedValue, useLoop} from "@shopify/react-native-skia";
export const AnimatedCircle = () => {
const size = 256;
const r = size * 0.33;
const progress = useLoop({ duration: 2000 });
const scale = useComputedValue(() => 0.5 + progress.current * 0.5, [progress]);
return (
<Canvas style={{ flex: 1 }}>
<Circle cx={r} cy={r} r={r} color="lightblue" transform={[{scale}]} />
</Canvas>
);
};
This code creates a circle that scales up and down continuously. The useLoop
hook provides a looping animation value, which we use to compute the scale of our circle.
Advanced Usage
React Native Skia offers advanced features for more complex graphics and animations.
Path Drawing
You can create complex shapes using paths:
import {Canvas, Path, Skia} from "@shopify/react-native-skia";
export const StarPath = () => {
const star = Skia.Path.MakeFromSVGString(
"M 128 0 L 168 80 L 256 93 L 192 155 L 207 244 L 128 202 L 49 244 L 64 155 L 0 93 L 88 80 L 128 0 Z"
);
return (
<Canvas style={{ flex: 1 }}>
<Path path={star} color="gold" />
</Canvas>
);
};
This example draws a star shape using an SVG path string. The Skia.Path.MakeFromSVGString
method converts the SVG path to a Skia path object.
Gradients and Effects
React Native Skia supports various gradients and effects:
import {Canvas, Rect, LinearGradient, vec} from "@shopify/react-native-skia";
export const GradientRect = () => {
const width = 256;
const height = 256;
return (
<Canvas style={{ flex: 1 }}>
<Rect x={0} y={0} width={width} height={height}>
<LinearGradient
start={vec(0, 0)}
end={vec(width, height)}
colors={["#00ff87", "#60efff"]}
/>
</Rect>
</Canvas>
);
};
This code creates a rectangle filled with a linear gradient. The LinearGradient
component defines the gradient’s properties, including start and end points, and colors.
Conclusion
React Native Skia opens up a world of possibilities for creating visually stunning and performant 2D graphics in React Native applications. From simple shapes to complex animations and effects, this library provides the tools you need to bring your creative visions to life.
By leveraging the power of the Skia graphics engine, you can create smooth, responsive, and visually appealing user interfaces that work consistently across platforms. Whether you’re building games, data visualizations, or just want to add some flair to your app’s UI, React Native Skia is an excellent choice.
As you continue your journey with React Native Skia, you might find it helpful to explore related topics. Check out our articles on Framer Motion for React animations and React Native Elements for UI components to further enhance your React Native development skills.
Remember, the key to mastering React Native Skia is practice and experimentation. Don’t hesitate to push the boundaries and see what amazing graphics you can create!