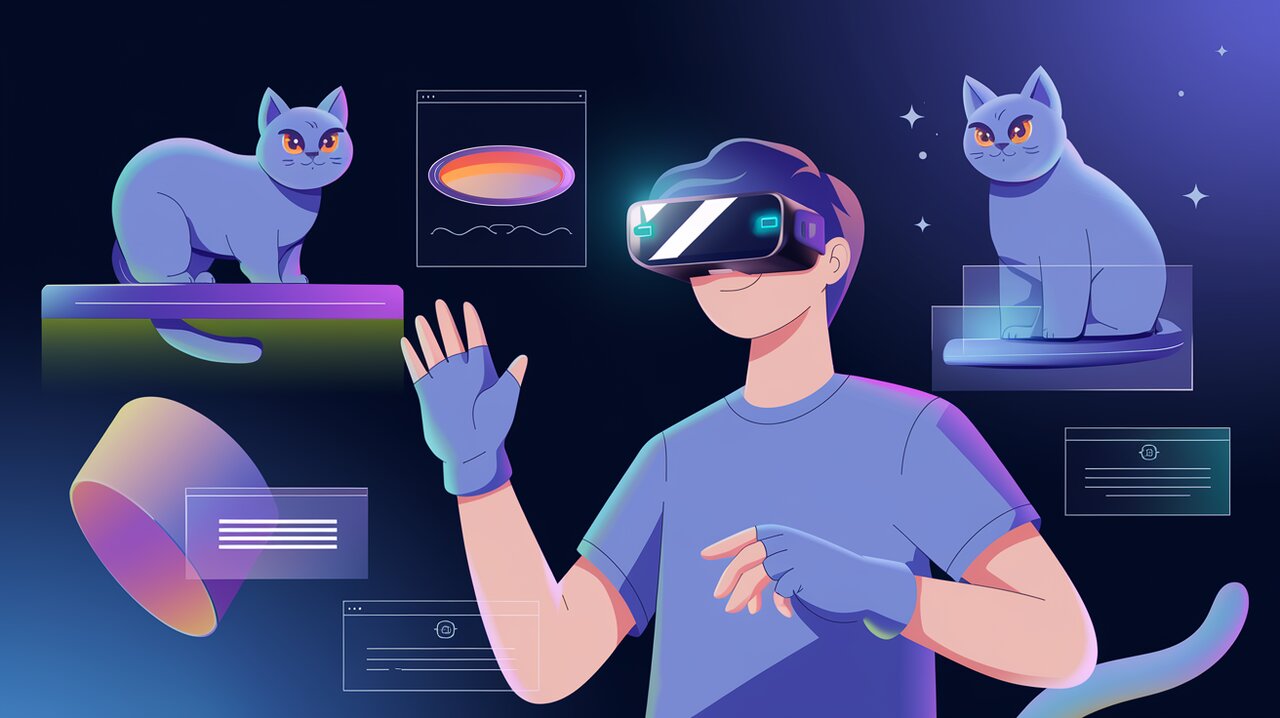
XR Odyssey: Embark on an Immersive Journey with @react-three/xr
Embark on an exhilarating journey into the realm of extended reality (XR) with @react-three/xr, a powerful library that seamlessly transforms your React Three Fiber applications into immersive experiences. Whether you’re crafting augmented reality (AR) or virtual reality (VR) content, this library provides the tools to create interactive and engaging XR environments with ease.
Unveiling the XR Toolkit
@react-three/xr is designed to work harmoniously with React Three Fiber, extending its capabilities to support WebXR applications. It provides a set of components and hooks that simplify the process of creating XR experiences, allowing developers to focus on crafting compelling content rather than wrestling with low-level XR APIs.
Setting Up Your XR Adventure
To begin your XR odyssey, you’ll need to install the necessary dependencies:
npm install three @react-three/fiber @react-three/xr@latest
With these tools at your disposal, you’re ready to create immersive experiences that blur the line between the digital and physical worlds.
Crafting Your First XR Scene
Let’s dive into a simple example that showcases the power of @react-three/xr:
import { Canvas } from '@react-three/fiber'
import { XR, createXRStore } from '@react-three/xr'
import { useState } from 'react'
const store = createXRStore()
export function App() {
const [red, setRed] = useState(false)
return (
<>
<button onClick={() => store.enterAR()}>Enter AR</button>
<Canvas>
<XR store={store}>
<mesh
pointerEventsType={{ deny: 'grab' }}
onClick={() => setRed(!red)}
position={[0, 1, -1]}
>
<boxGeometry />
<meshBasicMaterial color={red ? 'red' : 'blue'} />
</mesh>
</XR>
</Canvas>
</>
)
}
This example creates a simple AR scene with a color-changing cube. Let’s break down the key components:
- We create an XR store using
createXRStore()
. - The
XR
component wraps our 3D content, enabling XR functionality. - A button triggers the
enterAR()
function to initiate the AR experience. - The mesh responds to user interaction, changing color when clicked.
Navigating the XR Landscape
@react-three/xr offers a rich set of features to enhance your XR applications:
- Interaction Handling: The library provides intuitive ways to handle user interactions in XR environments. You can easily respond to touches, pointing, and other XR-specific input methods.
- Custom Controllers and Hands: Tailor the appearance and behavior of controllers and hands in your XR scene. This allows for more immersive and natural interactions within your application.
- Teleportation: Implement smooth teleportation mechanics for VR experiences, allowing users to navigate large virtual spaces comfortably.
- Object Detection: Leverage AR capabilities to detect real-world objects and integrate them into your virtual scene, creating a seamless blend of reality and digital content.
- Anchors: Use anchors to persistently place virtual objects in the real world, ensuring they maintain their position across AR sessions.
- Hit Testing: Perform hit tests to accurately place virtual objects on real-world surfaces, enhancing the realism of your AR applications.
Advanced XR Techniques
As you become more comfortable with @react-three/xr, you can explore advanced features to create even more compelling experiences:
Layers and Compositing
Utilize XR layers for optimal performance and visual quality in your applications. This is particularly useful for rendering high-fidelity content in VR headsets.
import { XR, XRLayer } from '@react-three/xr'
function App() {
return (
<XR>
<XRLayer>
{/* High-performance content goes here */}
</XRLayer>
{/* Regular scene content */}
</XR>
)
}
DOM Overlays
Integrate 2D DOM elements into your XR scene for user interfaces that combine the best of web and XR technologies.
import { XR, DomOverlay } from '@react-three/xr'
function App() {
return (
<XR>
<DomOverlay>
<div style={{ position: 'absolute', top: 0, left: 0 }}>
<h1>Welcome to XR!</h1>
</div>
</DomOverlay>
{/* 3D scene content */}
</XR>
)
}
Custom Input Sources
Create tailored interactions for different XR input devices, enhancing the versatility of your application across various XR platforms.
import { XR, useXRInputSource } from '@react-three/xr'
function CustomController() {
const inputSource = useXRInputSource()
// Implement custom controller behavior
return <mesh>{/* Controller mesh */}</mesh>
}
Charting Your XR Course
As you continue your XR odyssey with @react-three/xr, consider exploring these related topics to expand your immersive development skills:
- Dive deeper into 3D graphics with React Three Fiber
- Enhance your animations with Framer Motion
- Optimize performance with React Window
Conclusion
@react-three/xr opens up a world of possibilities for creating immersive XR experiences using React and Three.js. By leveraging its powerful features and intuitive API, you can craft engaging AR and VR applications that push the boundaries of web technology. As the XR landscape continues to evolve, this library stands as a valuable tool in any developer’s arsenal, ready to bring imaginative digital worlds to life.
Embark on your XR odyssey today, and transform your ideas into captivating immersive experiences that will leave users in awe. The future of web interactivity awaits – are you ready to explore it?